In the world of development, errors are the inevitable part that needs proper handling for the efficient working of the code we develop. In Odoo 18, there are many kinds of errors and exceptions possible while running the module or code under development.
Error management in Odoo 18 involves understanding how the system handles exceptions and bugs, as well as employing good practices to manage and resolve them effectively.
During the process of bug tracking and management, the system needs to understand and trace back them from the bottom and resolve them.
Odoo 18 has common and standard exceptions like Attribute Error, Value Error, Type Error, Validation Error, etc
Raising Exceptions
The Odoo 18 exceptions are raised to ensure that the system behaves as expected under some conditions. The exceptions get triggered for various reasons, such as invalid user inputs, flow against the validation conditions, missing of records, etc. Based on that, there are various kinds of exceptions.
1. ValidationError
At any time, we can raise an exception in Odoo using the ValidationError based on any condition we need to set.
For example, we can just set a Validation error for the stock.picking model if the field partner_id, ie ‘Receive From’ is not filled using the code.
from odoo import models
from odoo.exceptions import ValidationError
class StockPicking(models.Model):
_inherit = 'stock.picking'
def button_validate(self):
result = super(StockPicking, self).button_validate()
if not self.partner_id:
raise ValidationError('Fill in the field "Receive From"')
return result
This, whenever we try to validate the picking without filing the ‘Receive From’ field, it shows like
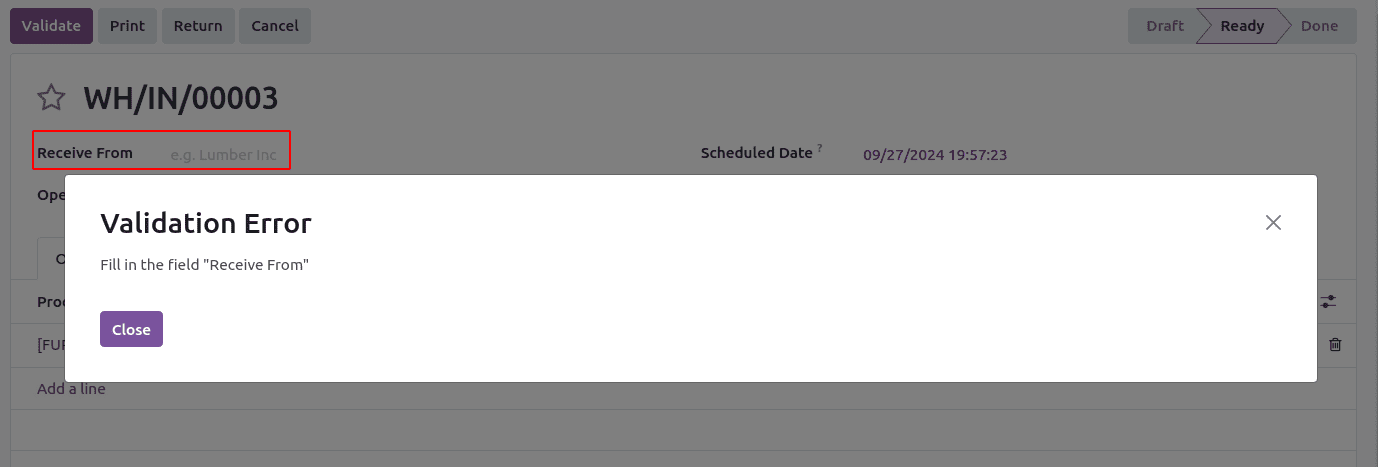
2. UserError
Similarly, User Errors can also be raised with the needed constraints or conditions with which the system undergoes checks for every record. This needs to import UserError from odoo.exceptions and we can just replace the previous code as below to demonstrate the workflow.
from odoo import models
from odoo.exceptions import UserError
class StockPicking(models.Model):
_inherit = 'stock.picking'
def button_validate(self):
result = super(StockPicking, self).button_validate()
if not self.partner_id:
raise UserError('Fill in the field "Receive From"')
return result
And in the UI, whenever the condition is true, the exception will pop up like
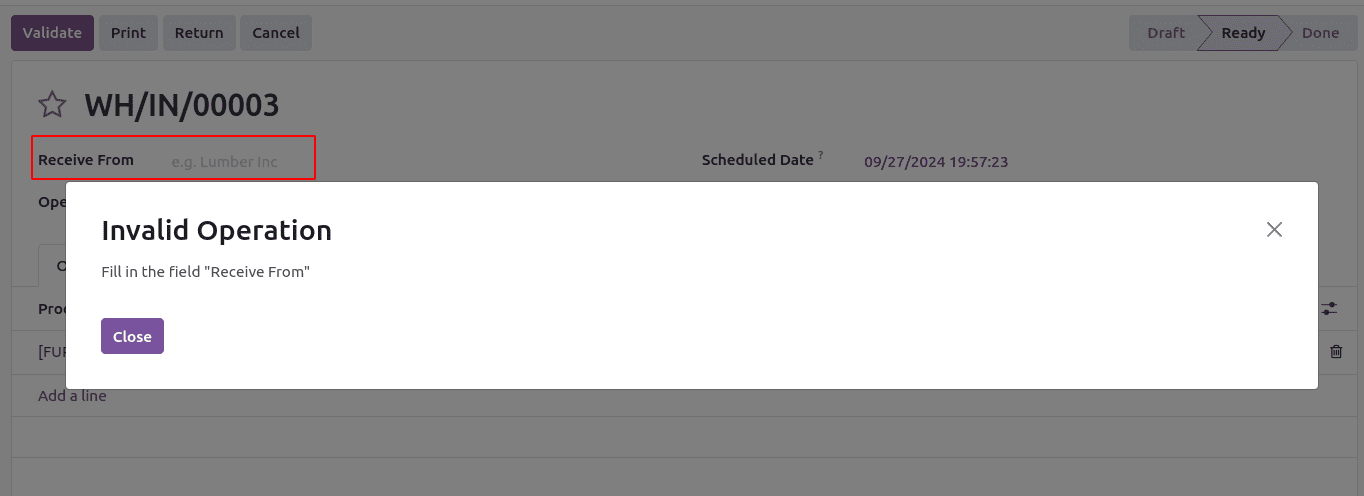
3. AccessError
Next, let’s check how the Access Denied Warning is running in Odoo 18. This exception is used when the user tries to log in to the Odoo instance with invalid login details. First, we need to import AccessError as
from odoo.exceptions import AccessError
Now, let’s write a simple Python code to block all the users other than Admin from accessing a document.
if not self.env.is_admin():
raise AccessError(_("Sorry, you are not allowed to access this document."))
4. MissingError
These Missing Errors are used in Odoo 18 usually when the user tries to access any record or data that is not actually available in the database of the instance. It may have either been deleted/ removed or doesn’t exist at all.
In the previous example code of validating a stock picking, let’s try to access any record with a random ID, say 1000 as
def button_validate(self):
result = super(StockPicking, self).button_validate()
print(self.browse(1000).name)
return result
Here, the stock.picking(1000,) is a non-existing record and hence, while clicking on the Validate button, it will pop up the Odoo 18’s inbuilt exception as
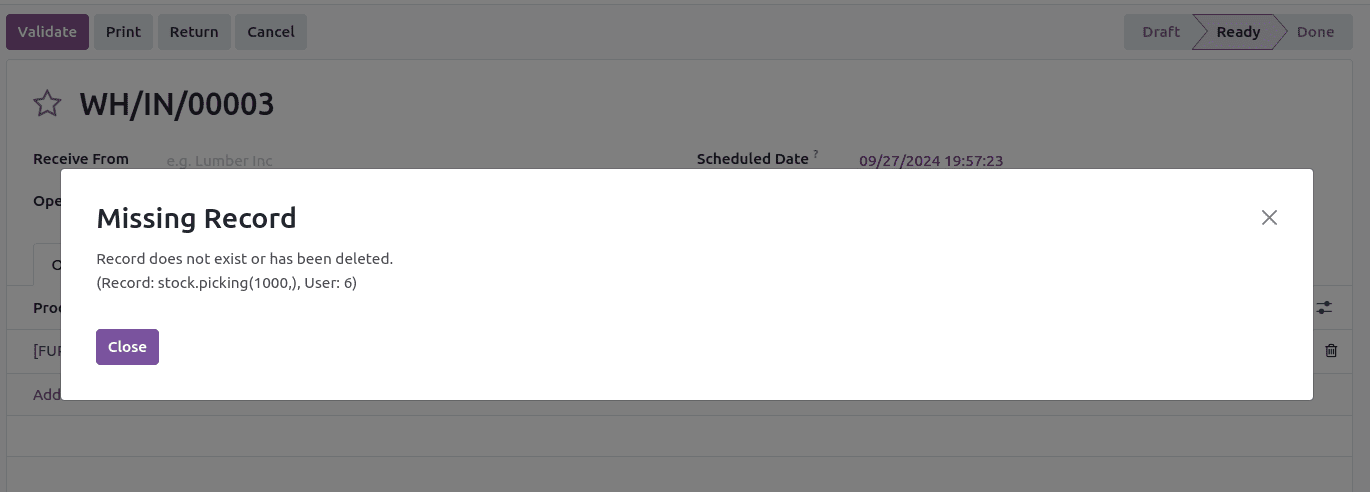
We can add our own MissingError exception first by importing it from odoo.exceptions and then by raising it as below.
from odoo import models
from odoo.exceptions import MissingError
class StockPicking(models.Model):
_inherit = 'stock.picking'
def button_validate(self):
raise MissingError('Missing Error Demo')
return super(StockPicking, self).button_validate()
It will simply raise a Missing Error as
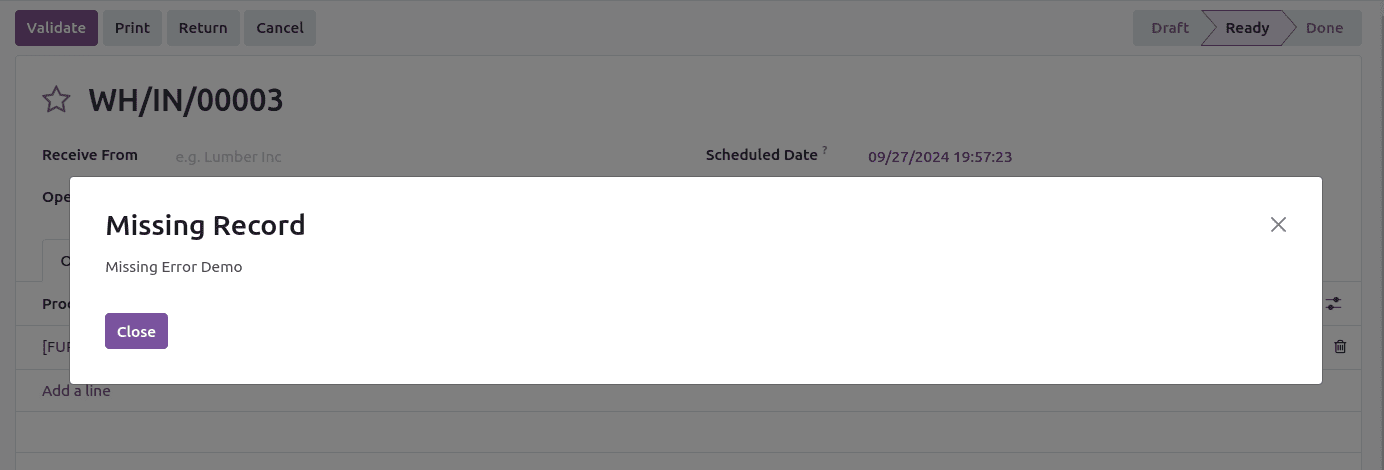
5. AccessDenied
Odoo usually makes use of this Access Denied exception in the case when a user tries to perform any kind of action or operation which he/she is not allowed to do due to the restriction in the access rights. These kinds of errors are quite common to use for maintaining security for the data and it can be done based on the user groups set for the concerned module.
For example,
from odoo import models
from odoo.exceptions import AccessDenied
class StockPicking(models.Model):
_inherit = 'stock.picking'
def button_validate(self):
if not self.env.is_admin():
raise AccessDenied("You are not allowed to validate the Operation")
return super(StockPicking, self).button_validate()
Here, for any user other than Admin, the right to validate the stock movement will be denied. Let us login to the system as any other internal user with no Admin access and try to validate the picking. The error will come like
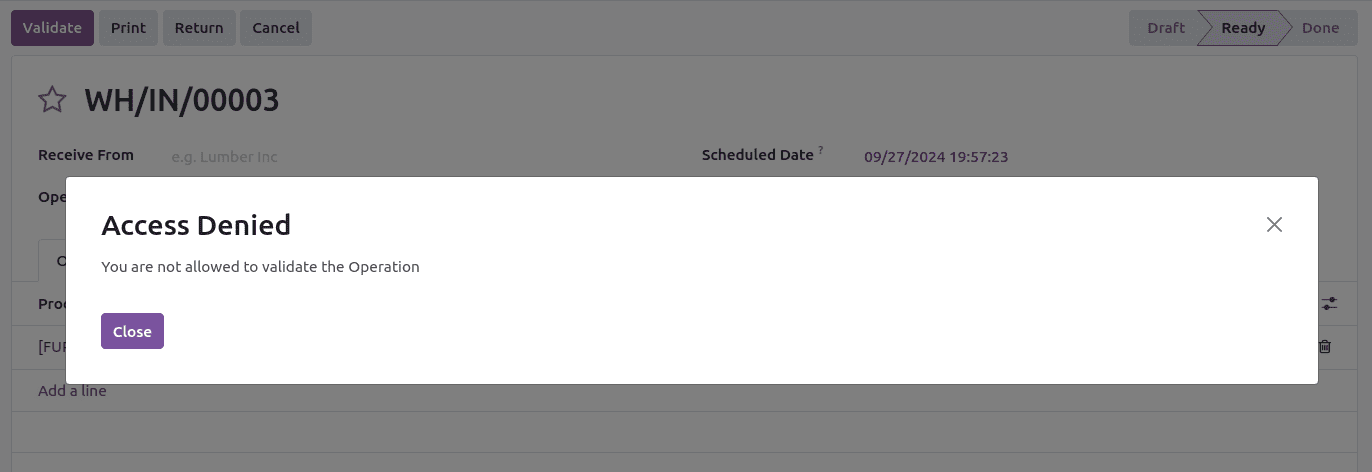
Insufficient Permissions, Misconfigured Access Control Lists (ACLs), Restricted Record Rules, Superuser Requirements, etc can be the causes of Access Denial.
6.CacheMiss
This is a kind of error that is raised by Odoo in the case when a record or data is not found. Odoo usually caches data about the record that has been accessed recently for some time. This CacheMiss occurs when the needed record is not found in the cache. It can be caused due to high traffic or the memory limits allowed.
country = self.env['res.country].browse(1)
print(country.name) # This access will hit the cache, no database query
Here, in the first line, the ‘country’ variable has a value. Odoo fetches it from the database and stores it in the cache. In the second line, ‘country.name’ is printed. During this time, Odoo will return it from the cache without requiring to run a database query again as in the first line.
In case if it is not found in the cache, a CacheMiss exception will pop up.
7. RedirectWarning
RedirectWarning can be used to redirect the workflow to a particular page instead of giving any warning to the user. That is, there can be needs such as the user needs to be redirected to another wizard or action. In such cases, RedirectWarning can be useful to suggest an alternate flow that should be followed, such as confirming an operation or redirecting to a form view before continuing with the original action.
The necessary parameters for RedirectWarning are
* action_id: It is the id of the action where to perform the redirection
* message: It is the message for the exception
* button_text: It is the text to put on the button that will trigger the redirection
With our previous example of validating a picking, we have made button_validate() method super, and let’s try to do a demo for the Redirect warning in that method as
from odoo import _, models
from odoo.exceptions import RedirectWarning
class StockPicking(models.Model):
_inherit = 'stock.picking'
def button_validate(self):
warehouse_action = self.env.ref('stock.action_warehouse_form')
msg = _('Please create a warehouse for company %s.',
self.env.company.display_name)
raise RedirectWarning(msg, warehouse_action.id, _('Go to Warehouses'))
return super(StockPicking, self).button_validate()
Here, while validating the picking, the system will generate a RedirectWarning with a message on the body and two buttons named ‘Go to Warehouses’ and ‘Close’. On clicking on the former one, the control goes to the page of warehouse creation/ already created warehouses. The exception will be as:
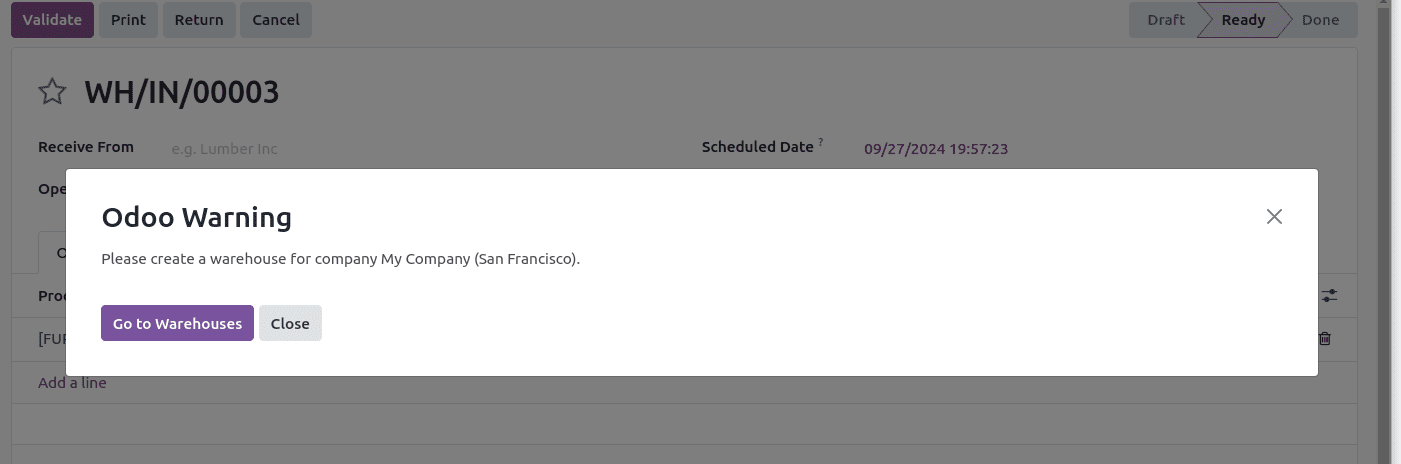
While clicking on the ‘Go to Warehouses’ button, we get the page as shown below.
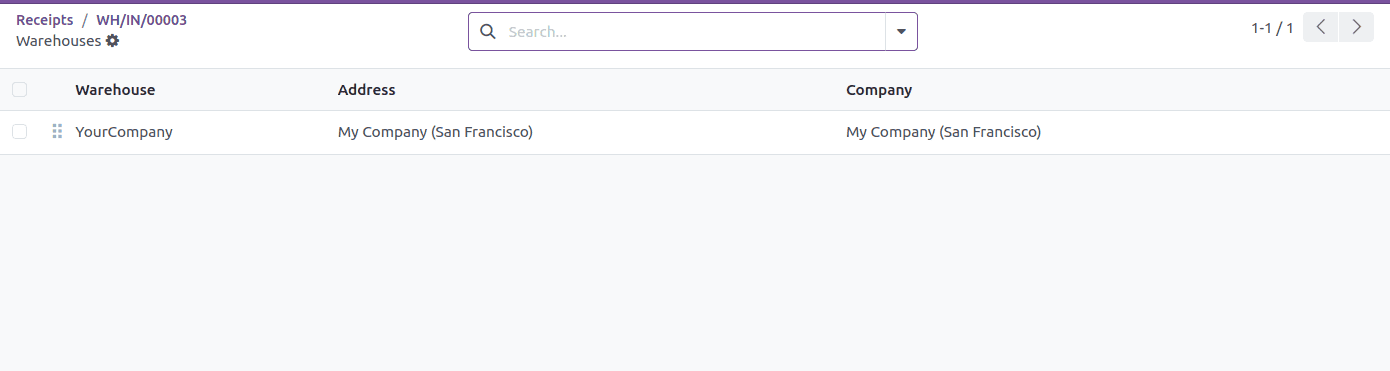
Odoo provides a comprehensive set of exceptions that developers can use to handle various scenarios, such as data validation issues, access control violations, records not found, or even complex workflows requiring redirection. Using these exceptions properly helps ensure that your application behaves predictably and provides clear feedback to users when something goes wrong.
To read more about What are the Expected Features in Odoo 18 ERP Software, refer to our blog What are the Expected Features in Odoo 18 ERP Software.