In today's digital age, sharing information seamlessly across different platforms and devices is essential for both personal and professional communication. Whether you're developing a chat application, a document management system, or a social networking app, enabling users to share files, links, and text is a fundamental feature that enhances the overall user experience.
In this blog post, we'll explore how to share files, links, and text using Flutter.
First, add a share icon to the body of your Flutter page. The code to implement this is provided below:
import 'package:flutter/material.dart';
class SharePage extends StatefulWidget {
const SharePage({super.key, required this.title});
final String title;
@override
State<SharePage> createState() => _SharePageState();
}
class _SharePageState extends State<SharePage> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Theme.of(context).colorScheme.inversePrimary,
title: Text(widget.title),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
InkWell(
child: Container(
padding: EdgeInsets.symmetric(horizontal: 20, vertical: 10),
child: Row(
mainAxisSize: MainAxisSize.min,
children: [
Icon(
Icons.share,
color: Colors.black,
),
SizedBox(width: 40),
Text(
'Share text',
style: TextStyle(
fontWeight: FontWeight.bold,
color: Colors.black,
fontSize: 19),
),
],
),
),
),
],
),
),
);
}
}
The output of the given code is:
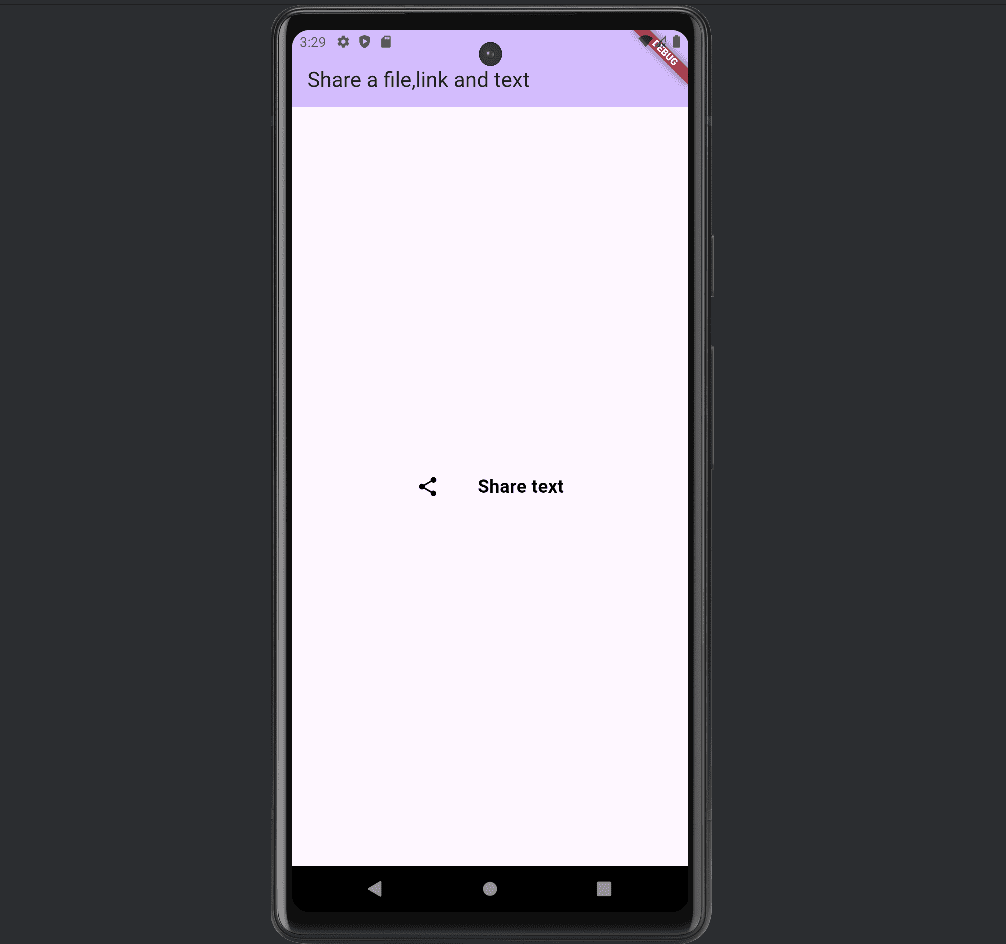
Next, implement the share functionality by importing the share_plus package as shown below:
import 'package:share_plus/share_plus.dart';
Now add ontap for InkWell as below code:
First, share the text as shown below:
Future<void> _shareText() async {
String message =
'Hello! Welcome to Cybrosys.';
Share.share(message, subject: 'Welcome Message');
}
The complete code is provided below:
import 'package:flutter/material.dart';
import 'package:share_plus/share_plus.dart';
class SharePage extends StatefulWidget {
const SharePage({super.key, required this.title});
final String title;
@override
State<SharePage> createState() => _SharePageState();
}
class _SharePageState extends State<SharePage> {
Future<void> _shareText() async {
String message =
'Hello! Welcome to Cybrosys.';
Share.share(message, subject: 'Welcome Message');
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Theme.of(context).colorScheme.inversePrimary,
title: Text(widget.title),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
InkWell(
onTap: _shareText,
child: Container(
padding: EdgeInsets.symmetric(horizontal: 20, vertical: 10),
child: Row(
mainAxisSize: MainAxisSize.min,
children: [
Icon(
Icons.share,
color: Colors.black,
),
SizedBox(width: 40),
Text(
'Share text',
style: TextStyle(
fontWeight: FontWeight.bold,
color: Colors.black,
fontSize: 19),
),
],
),
),
),
],
),
),
);
}
}
Clicking the share button will display the image below, where you can share the text via other apps:
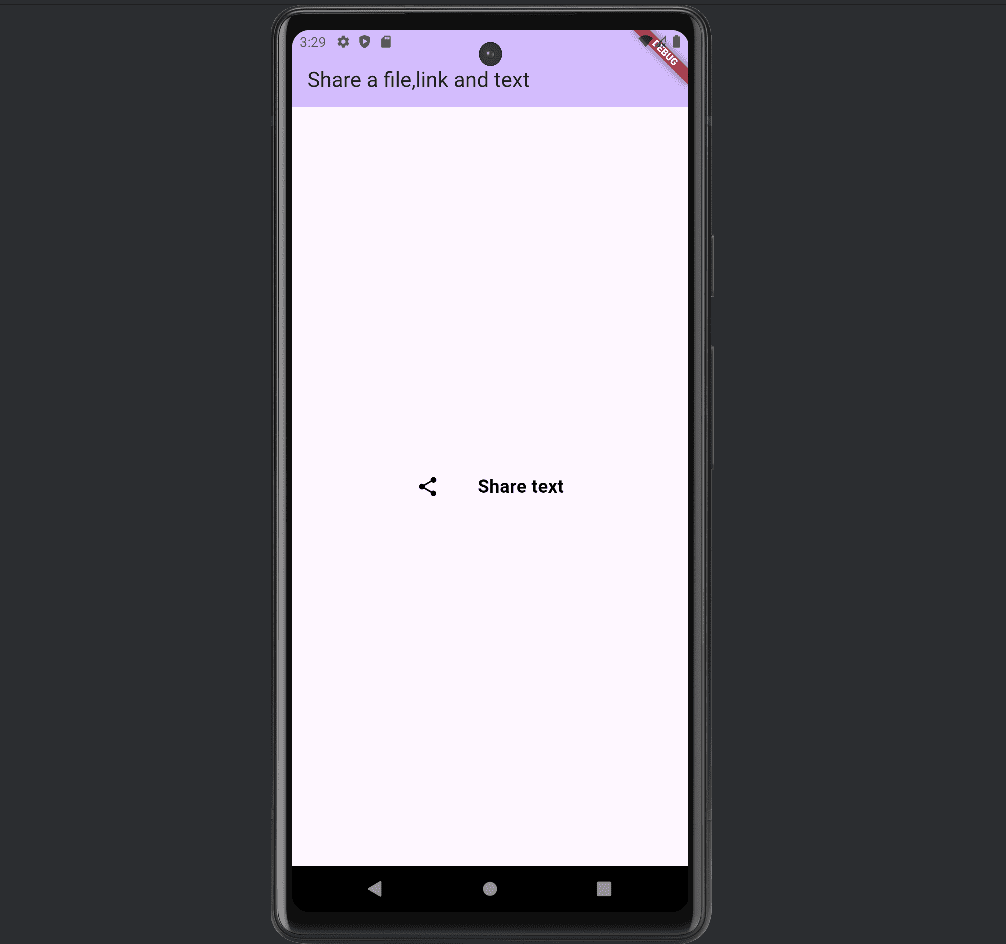
Now sharing the link as shown below:
Future<void> _shareLink() async {
String message =
'Hello! Welcome to Cybrosys. Follow the link to open Cybrosys Website: https://www.cybrosys.com/';
Share.share(message, subject: 'Welcome Message');
}
The complete code is as follows:
import 'package:flutter/material.dart';
import 'package:share_plus/share_plus.dart';
class SharePage extends StatefulWidget {
const SharePage({super.key, required this.title});
final String title;
@override
State<SharePage> createState() => _SharePageState();
}
class _SharePageState extends State<SharePage> {
Future<void> _shareLink() async {
String message =
'Hello! Welcome to Cybrosys. Follow the link to open Cybrosys Website: https://www.cybrosys.com/';
Share.share(message, subject: 'Welcome Message');
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Theme.of(context).colorScheme.inversePrimary,
title: Text(widget.title),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
InkWell(
onTap: _shareLink,
child: Container(
padding: EdgeInsets.symmetric(horizontal: 20, vertical: 10),
child: Row(
mainAxisSize: MainAxisSize.min,
children: [
Icon(
Icons.share,
color: Colors.black,
),
SizedBox(width: 40),
Text(
'Share Link',
style: TextStyle(
fontWeight: FontWeight.bold,
color: Colors.black,
fontSize: 19),
),
],
),
),
),
],
),
),
);
}
}
The output of the above code is shown in the image below, where you can share the link via other apps:
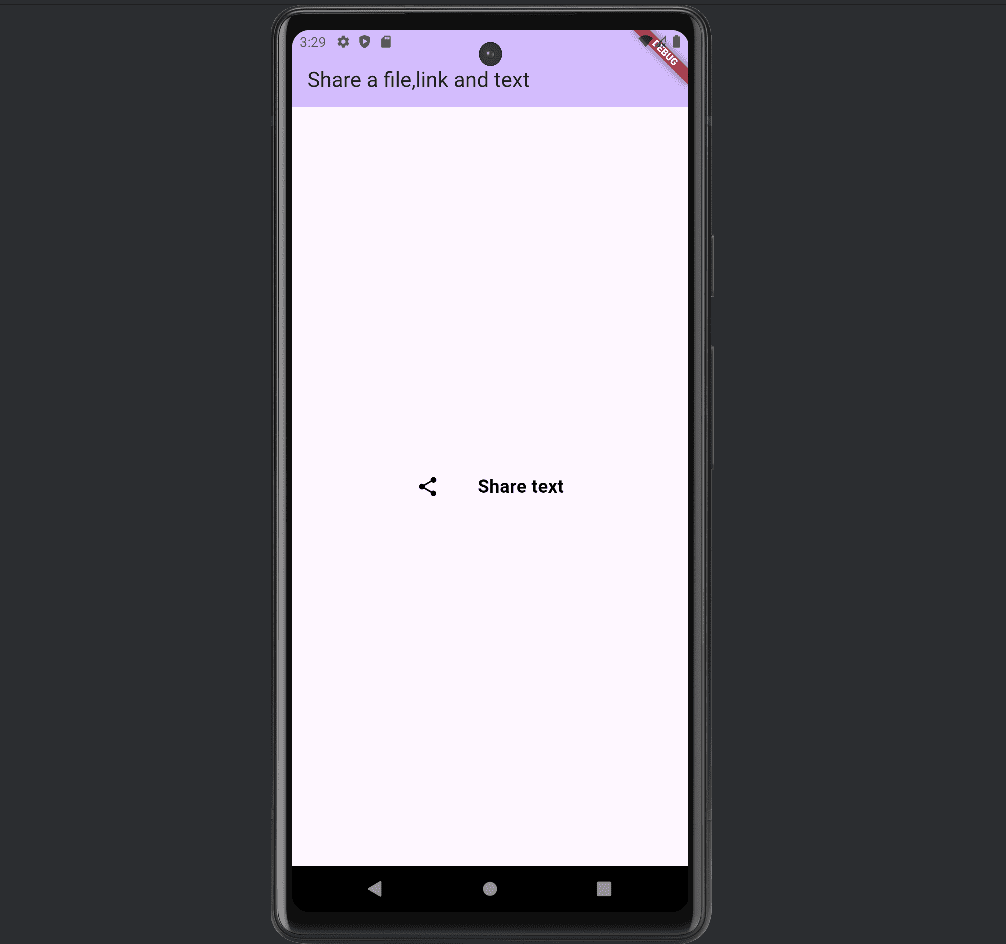
Now share the file. The following code will help to achieve this:
Future<void> _shareFile() async {
try {
final directory = await getApplicationDocumentsDirectory();
final File file = File('${directory.path}/example.txt');
if (!file.existsSync()) {
await file.writeAsString('This is an example file for sharing.');
}
Share.shareXFiles([XFile(file.path)], text: 'Sharing an example file');
} catch (e) {
print('error: $e');
}
}
final directory = await getApplicationDocumentsDirectory();
This line retrieves the directory where the application can store files. This is a platform-specific directory that you can write to.
final File file = File('${directory.path}/example.txt');
A File object is created with a path constructed from the application documents directory and a filename (example.txt).
if (!file.existsSync()) {
This condition checks if the file already exists. The method existsSync returns true if the file exists and false otherwise.
await file.writeAsString('This is an example file for sharing.');
If the file does not exist, this line writes a string ('This is an example file for sharing.') to the file. This operation is asynchronous, hence the await keyword.
And finally, the file can be shared by clicking the share button using the share_plus package as below:
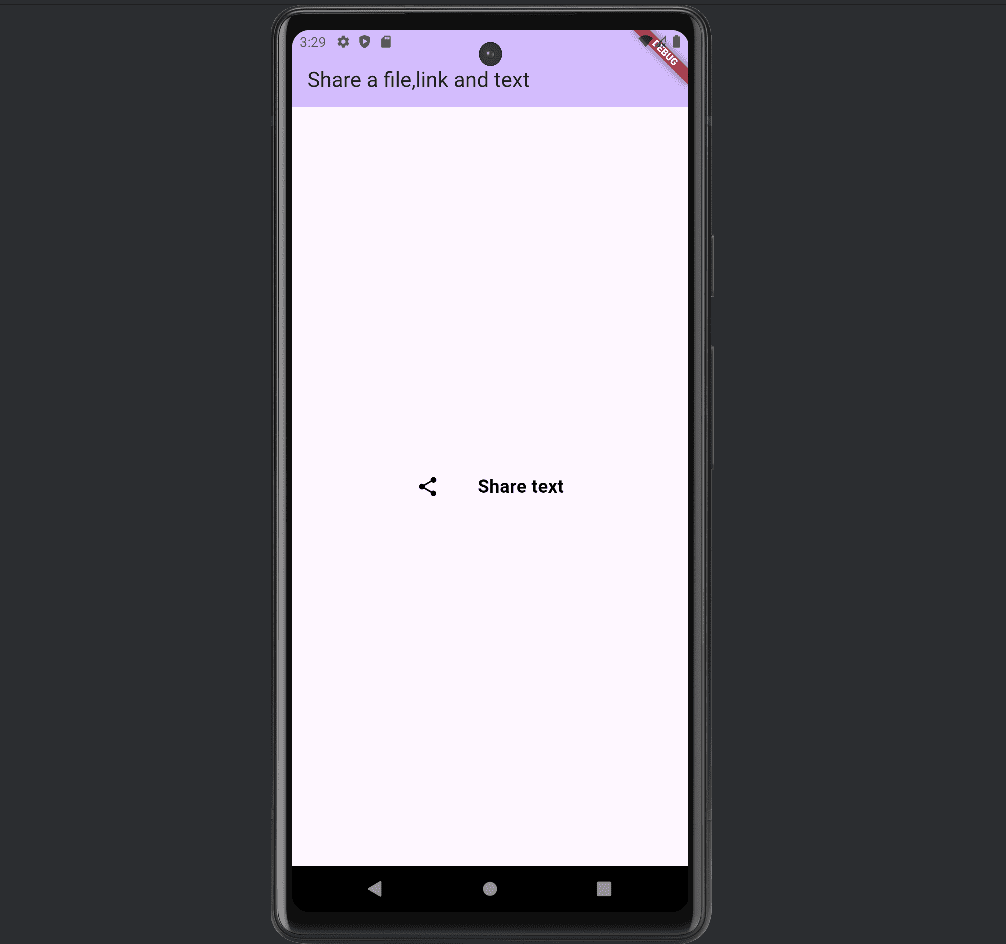
Sharing files, links, and text in a Flutter application can greatly enhance user experience by enabling seamless interaction with other apps and platforms. By integrating the share_plus package, you can easily implement share functionality to send content such as text, links, and files from your app.
To read more about How to Download & View a PDF File by Clicking the Notification in Flutter, refer to our blog How to Download & View a PDF File by Clicking the Notification in Flutter.