Odoo offers various view types, including Kanban, form, graph, tree, pivot, search, and calendar, to improve usability by displaying records in different, user-friendly formats.
In this blog, we will explore how to create a list view and the various attributes that can be utilized to improve its performance.
1. Create a new model class.teacher and declare its fields.
# -*- coding: utf-8 -*-
from odoo import api, fields, models, _
class ClassTeacher(models.Model):
_name = 'class.teacher'
_description = 'Class Teacher'
name = fields.Char(string='Name')
age = fields.Float(string='Age')
email = fields.Char(string='Email')
subject = fields.Char(string='Subject')
qualification = fields.Char(string='Qualification')
experience = fields.Float(string='Experience')
state = fields.Selection(string='State'
[('draft', 'Draft'),('confirm', 'Confirmed')],)
joining_date = fields.Date(string='Joining Date')
2. Create action for class.teacher
In Odoo 18, there is a change in root element. We can use ‘list’ instead of ‘tree’.
<record id="student_action_teacher" model="ir.actions.act_window">
<field name="name">Teachers</field>
<field name="type">ir.actions.act_window</field>
<field name="res_model">class.teacher</field>
<field name="view_mode">list,form</field>
</record>
3. Create view records
We can now add a new record to the ir_ui_view model by providing the ID, name, and the related model for which the view is created, as well as defining its layout. In the architecture section, we can specify the fields using the <list> tag.
<record id="class_teacher_view_list" model="ir.ui.view">
<field name="name">class.teacher.view.list</field>
<field name="model">class.teacher</field>
<field name="arch" type="xml">
<list>
<field name='name'/>
<field name='age'/>
<field name='email'/>
<field name='joining_date'/>
<field name='subject'/>
<field name='qualification'/>
<field name="state"/>
</list>
</field>
</record>
Now we get the following view,
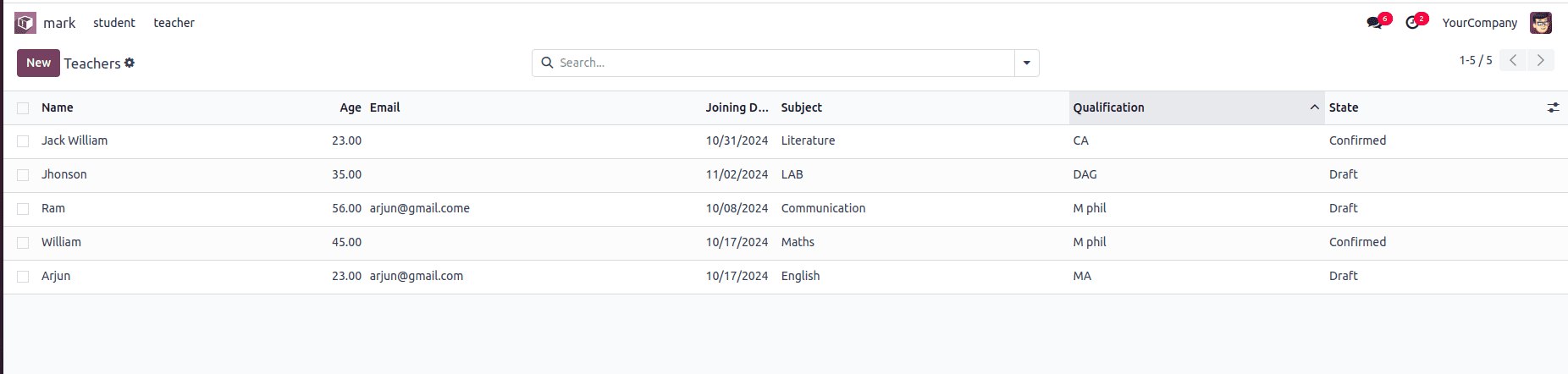
List root attributes:
1. String(str): The view title is shown only when you open an action without a name that targets a new dialog.
<list string="Teachers">
2. Create(bool): Allow or restrict record creation in the view and the default is True.
<list string="Teachers" create="0">
Now user can’t create record from the list view.
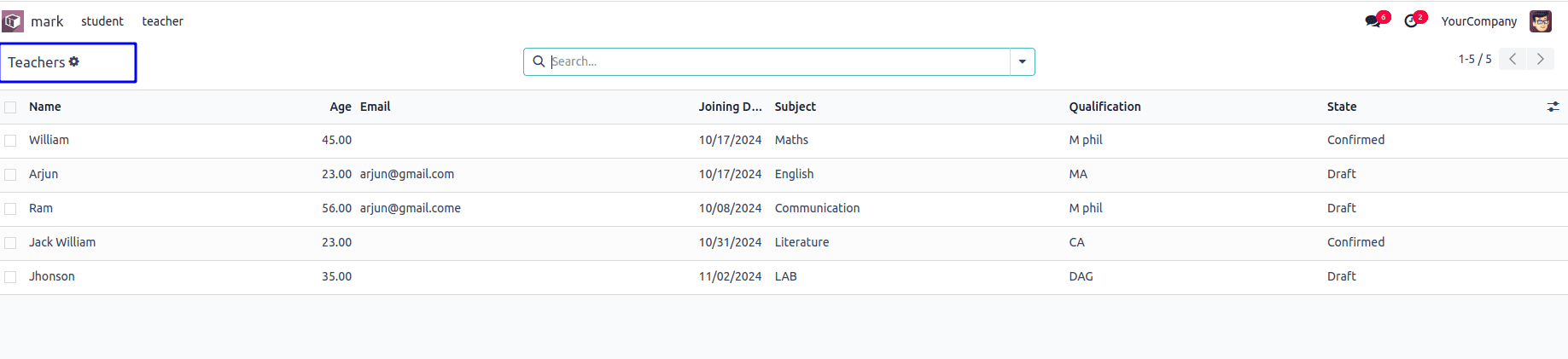
3. edit(bool): Allow or restrict record editing in the view and the default is True.
<list string="Teachers" edit="0">
4. delete(bool): Allow or prevent record deletion in the view via the Action dropdown.
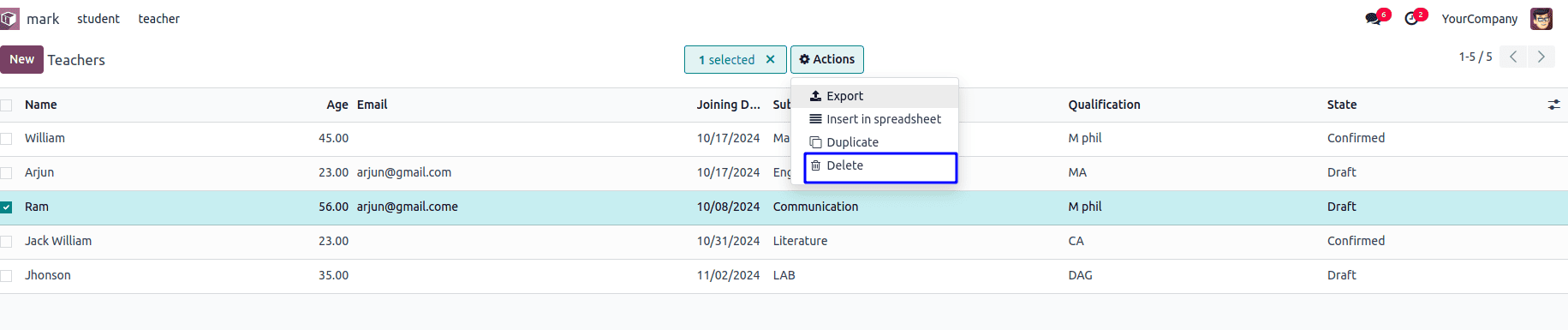
<list string="Teachers" delete="0">
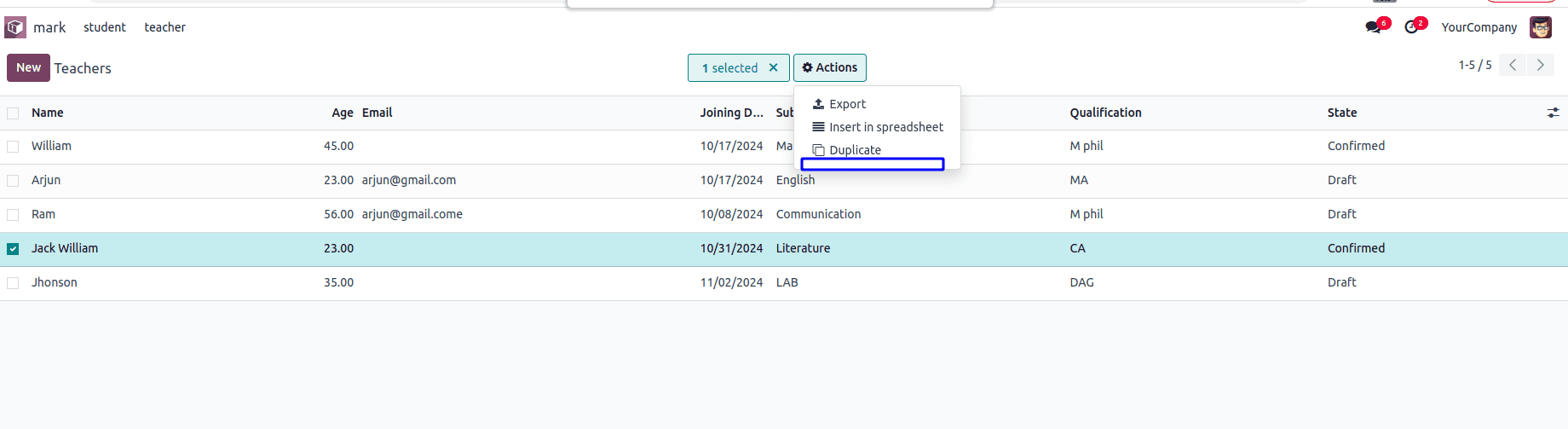
5. import(bool): Allow or prevent record importing in the list view.
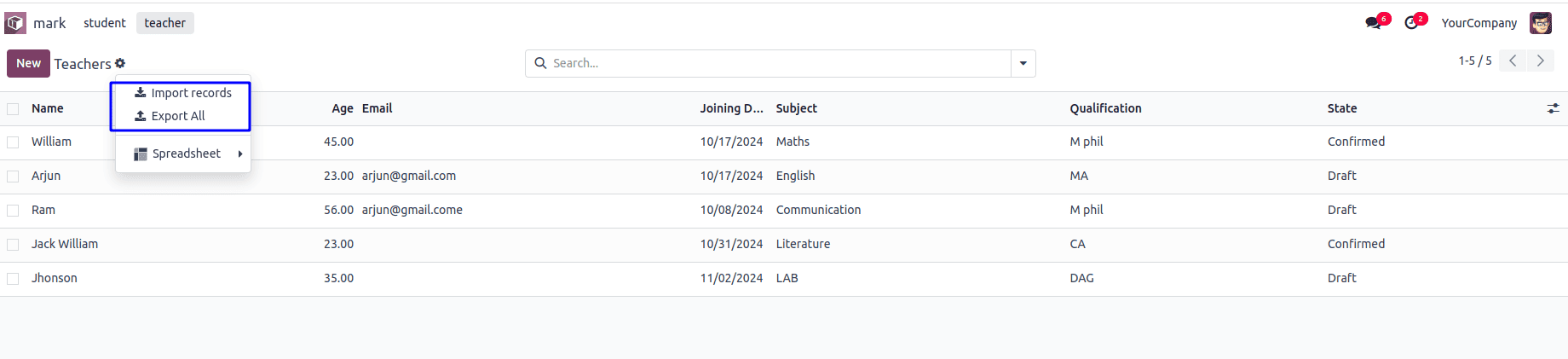
<list string="Teachers" import="0">
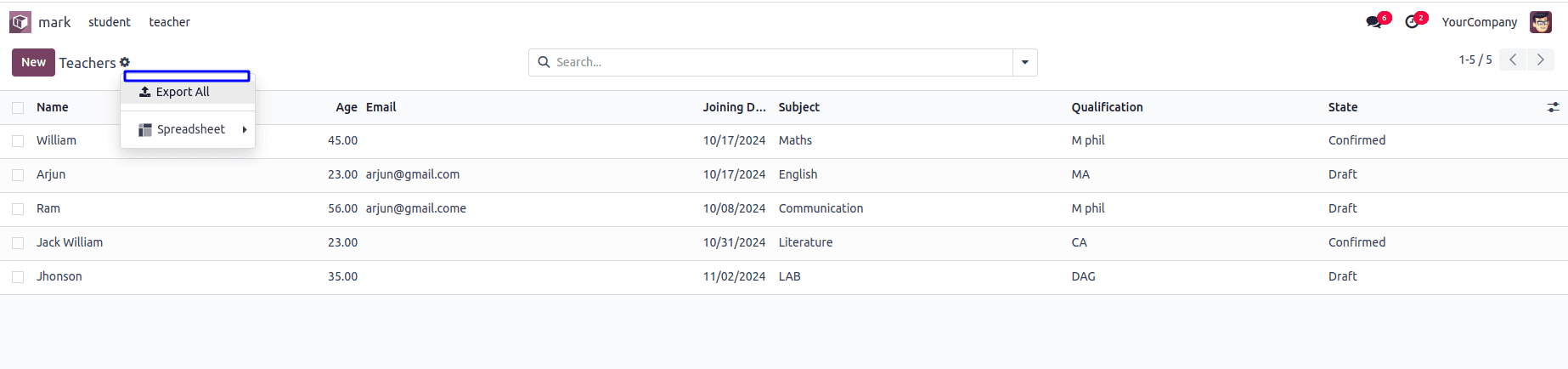
6.export_xlsx(bool): Allow or prevent record exporting in the list view.
<list string="Teachers" import="0" export_xlsx="0">
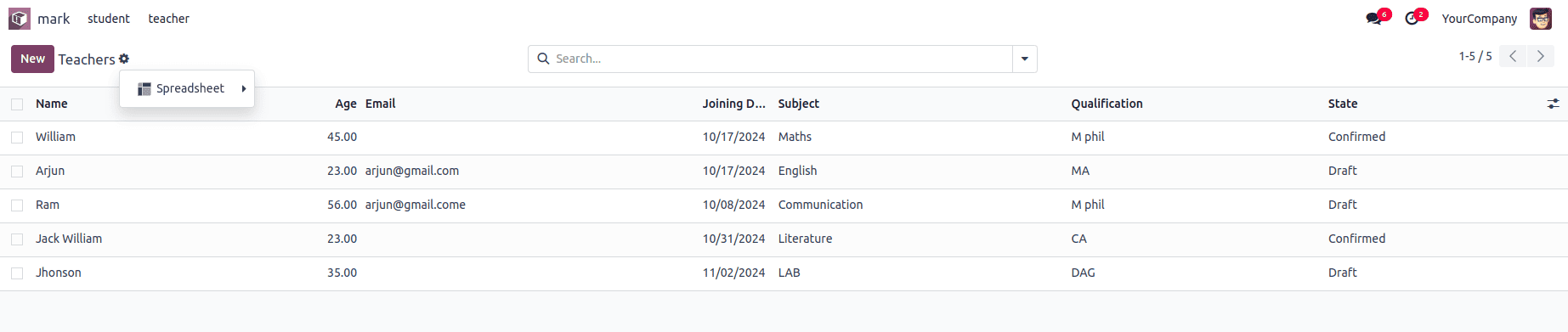
7. editable(str): Enable in-place editing of the view's records and allow the creation of new records directly from a row in the list.
This can have two possible options:
top: New records are created at the top of the list.
bottom: New records are created at the bottom of the list.
<list string="Teachers" editable="bottom">
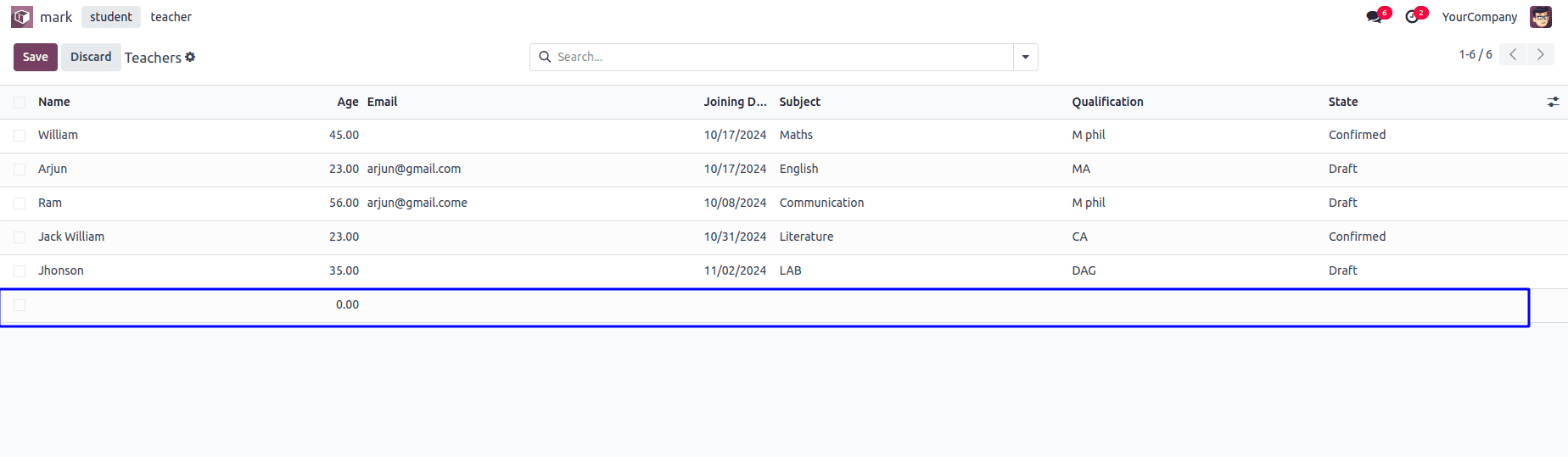
<list string="Teachers" editable="top">
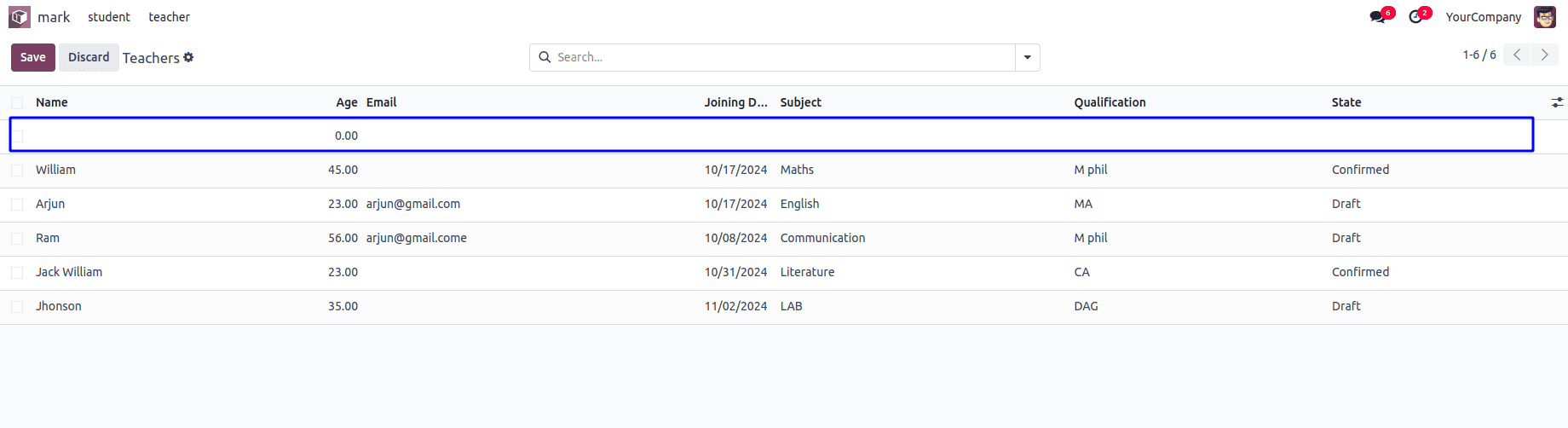
8. multi_edit(str): Enable batch editing to apply a single field value across multiple records simultaneously.
<list string="Teachers" multi_edit="1">
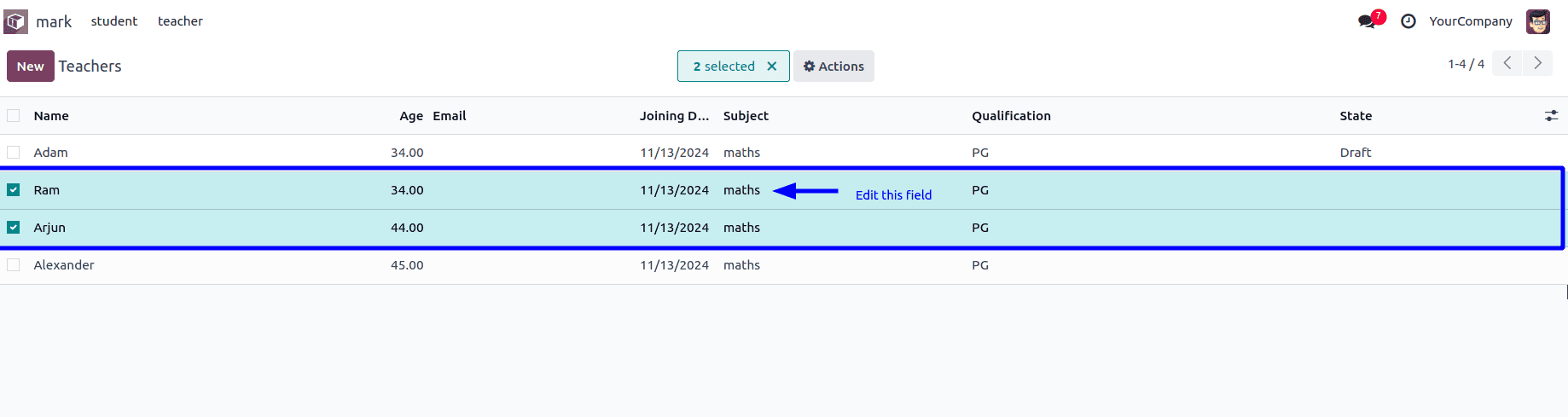
When we edit the field and enter a value, a confirmation dialog box appears as shown below.
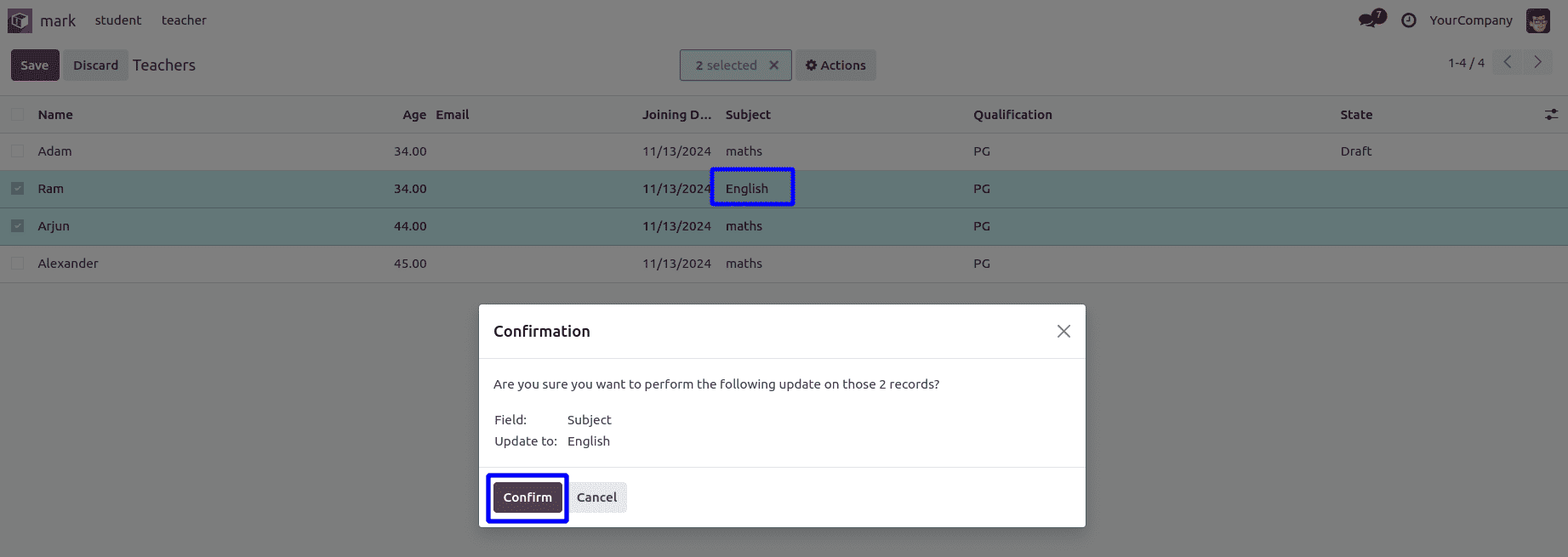
Upon clicking the confirm button, the selected records are updated as shown in the image below.
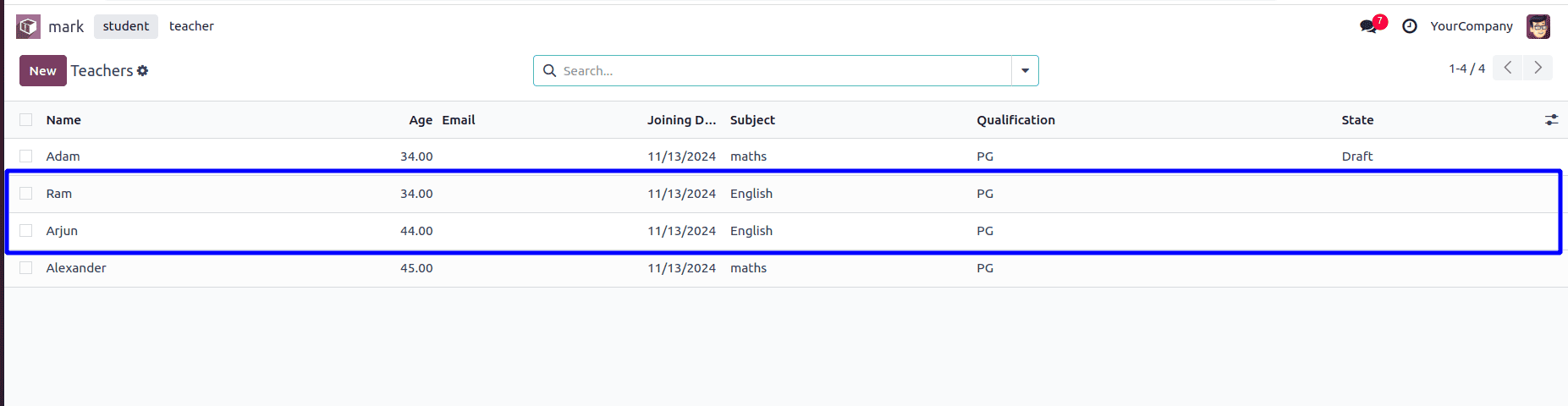
9. open_form_view(bool): Add a button at the end of each row to open the record in form view.
<list string="Teachers" editable="bottom" open_form_view="1">
It has effect only if the view is editable.
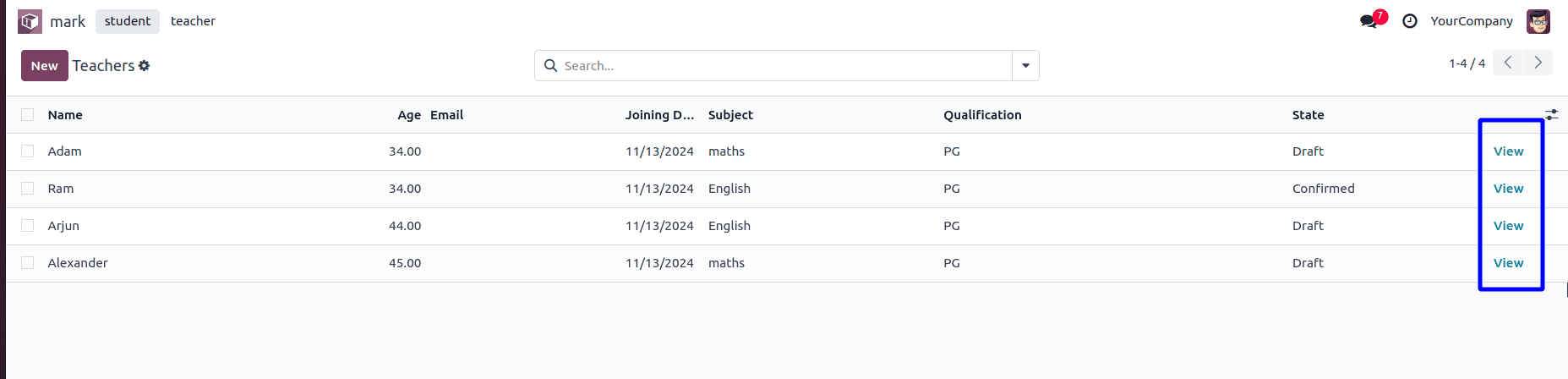
10. default_group_by(str): The field name to group records by default when no grouping is specified in the action or current search.
<list string="Teachers" default_group_by="state">

11. expand(bool): Indicates whether the first level of groups should be expanded by default when the list view is grouped.
<list string="Teachers" default_group_by="state" expand="1">
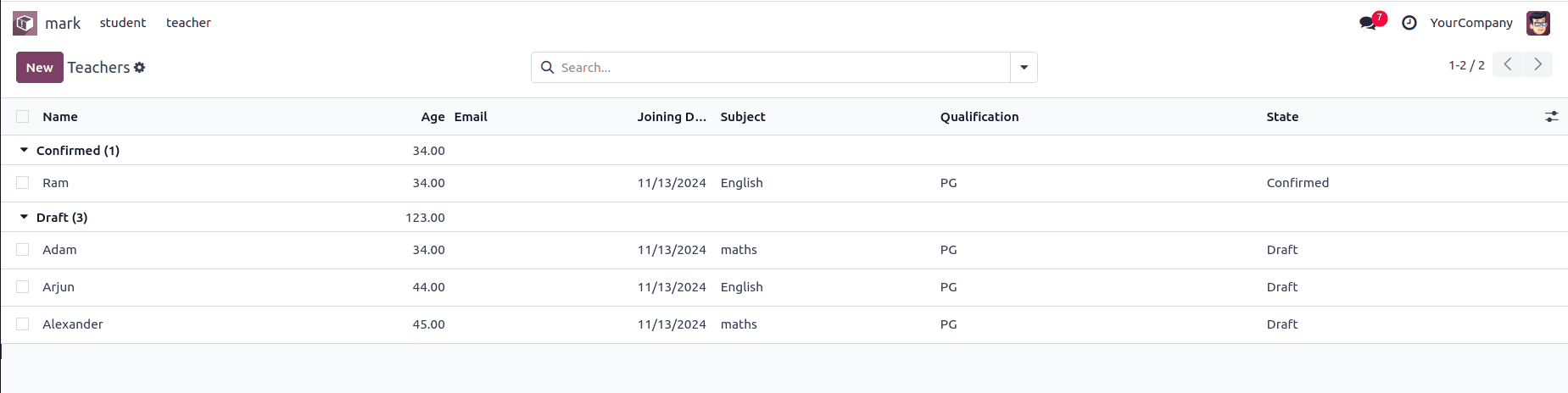
12. default_order(str): A list of field names, separated by commas, that overrides the ordering set by the model's _order attribute.
<list string="Teachers" default_order="age desc">
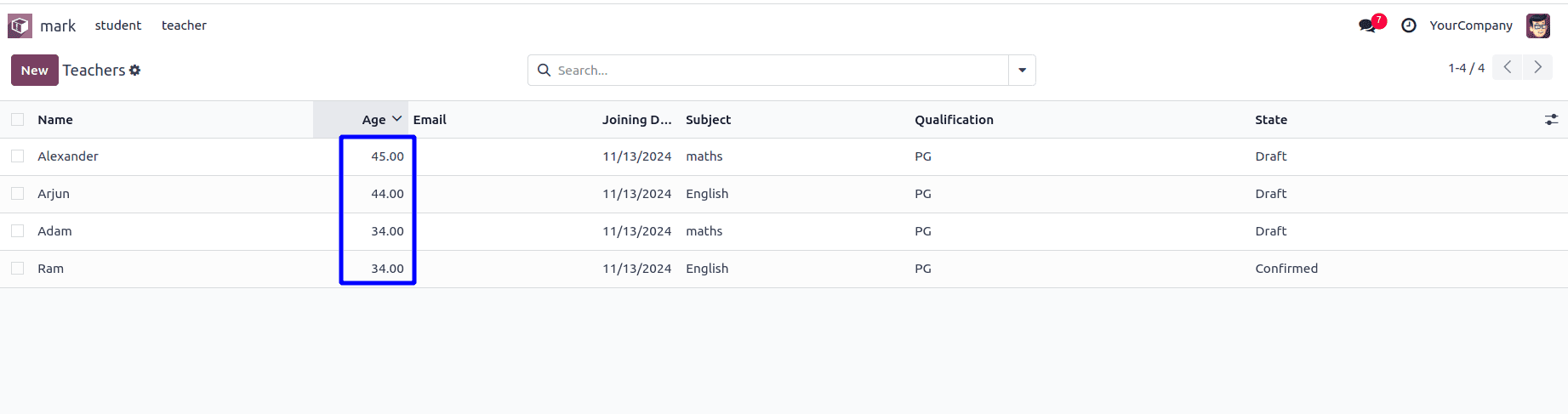
13. limit(int): The number of records on a page, by default it is 80 for the list and 4o for x2many.
<list string="Teachers" limit="2">
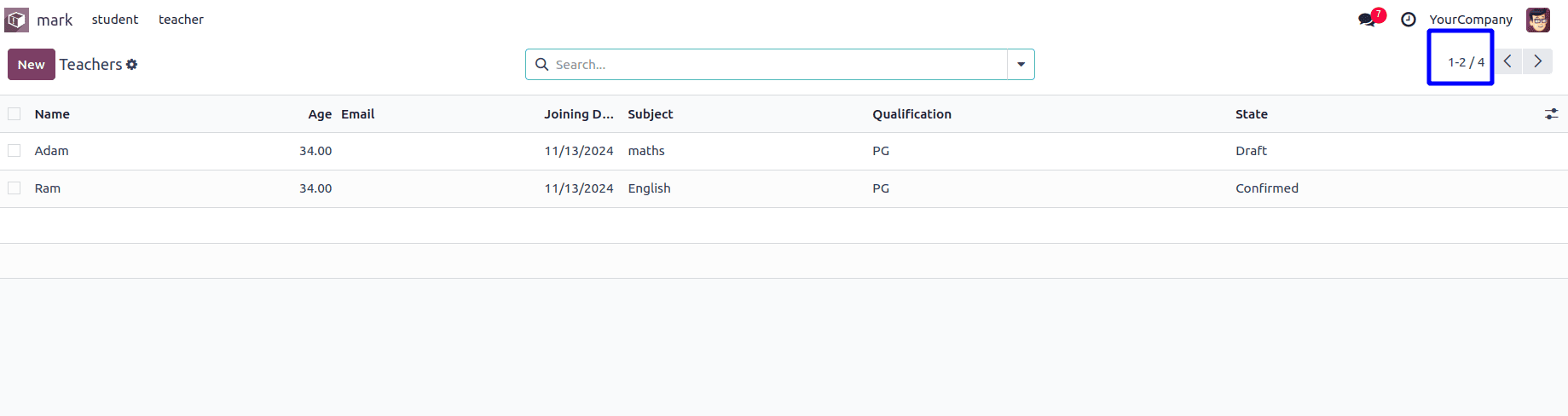
14. groups_limit(int): The default number of groups in list view.
<list string="Teachers" default_group_by="age" group_limit="2">
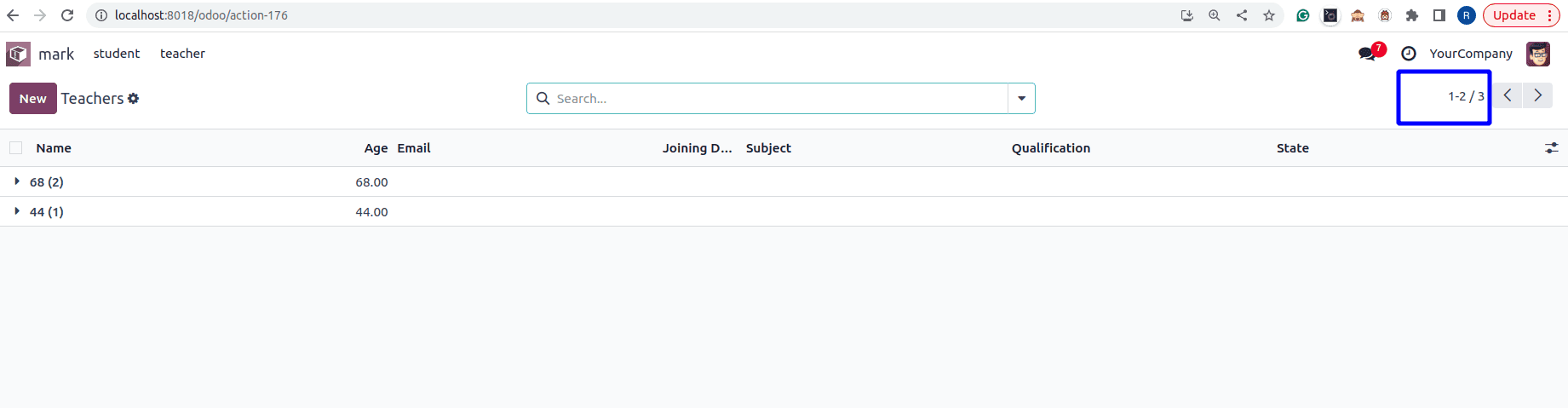
The screenshot shows only two groups displayed. To view the additional groups, click the right arrow indicated in the screenshot.
15. decoration-<style>: The decoration style should be applied to records based on the condition.
* decoration-it – ITALICS
* decoration-bf – BOLD
* decoration-danger – LIGHT RED
* decoration-primary – LIGHT PURPLE
* decoration-info – LIGHT BLUE
* decoration-warning – LIGHT BROWN
* decoration-muted – LIGHT GRAY
<list string="Teachers" decoration-success="state == 'confirm'" decoration-info="state == 'draft'">
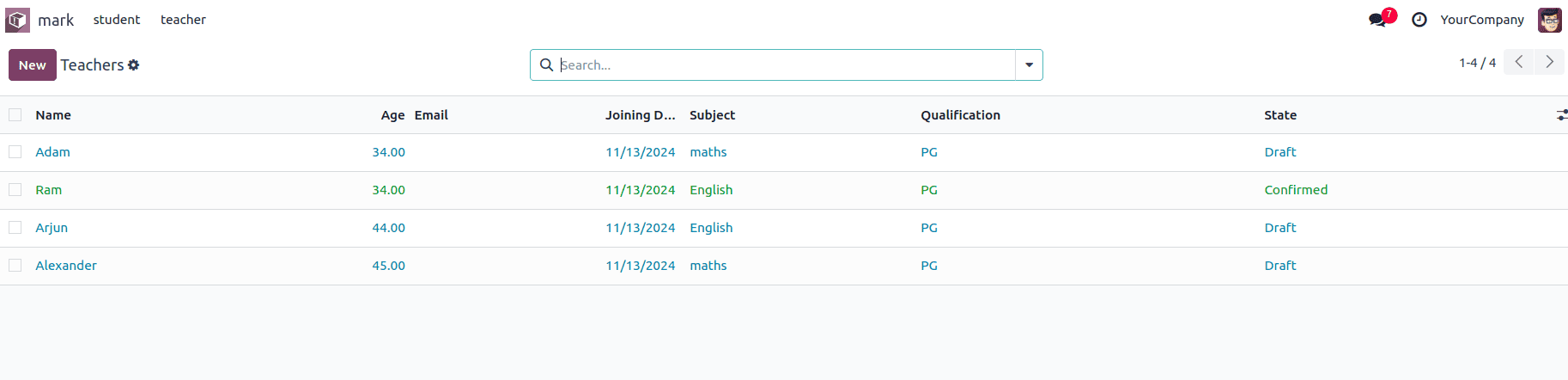
We can easily differentiate the records based on colors.
In summary, the ListView in the Odoo platform is among the most effective views available. It offers users a comprehensive overview of all records and can be customized to align with the operational requirements of the business.
To read more about List (Tree) View Decoration Attributes in Odoo 18, refer to our blog List (Tree) View Decoration Attributes in Odoo 18.