QR codes have gained widespread popularity as a convenient tool for sharing information, making payments, or connecting to networks with a simple scan. As a Flutter developer, incorporating QR code generation into your app can improve the user experience by enabling seamless data exchange. Whether you're working on an e-commerce application, an event registration platform, or a contactless solution, Flutter provides a number of libraries to efficiently generate QR codes.
In this blog, we'll explore how to generate a QR code using the qr_flutter package in a Flutter application.
Firstly, you need to import qr_flutter as below:
import 'package:qr_flutter/qr_flutter.dart';
Now add a field to enter text and button to the view as code below:
import 'package:flutter/material.dart';
class QrGeneratePage extends StatefulWidget {
@override
State<QrGeneratePage> createState() => _QrGeneratePageState();
}
class _QrGeneratePageState extends State<QrGeneratePage> {
TextEditingController controller =
TextEditingController();
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Generate QR Code',
style: TextStyle(
color: Colors.white,
fontWeight: FontWeight.bold,
),
),
backgroundColor: Colors.green,
),
body: Padding(
padding: const EdgeInsets.all(16.0),
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
TextField(
controller: controller,
decoration: const InputDecoration(
labelText: 'Enter text for QR Code',
border: OutlineInputBorder(),
),
),
const SizedBox(height: 20),
ElevatedButton(
onPressed: () {
},
child: const Text('Generate QR Code'),
),
],
),
),
);
}
}
The output of the above code is given below:
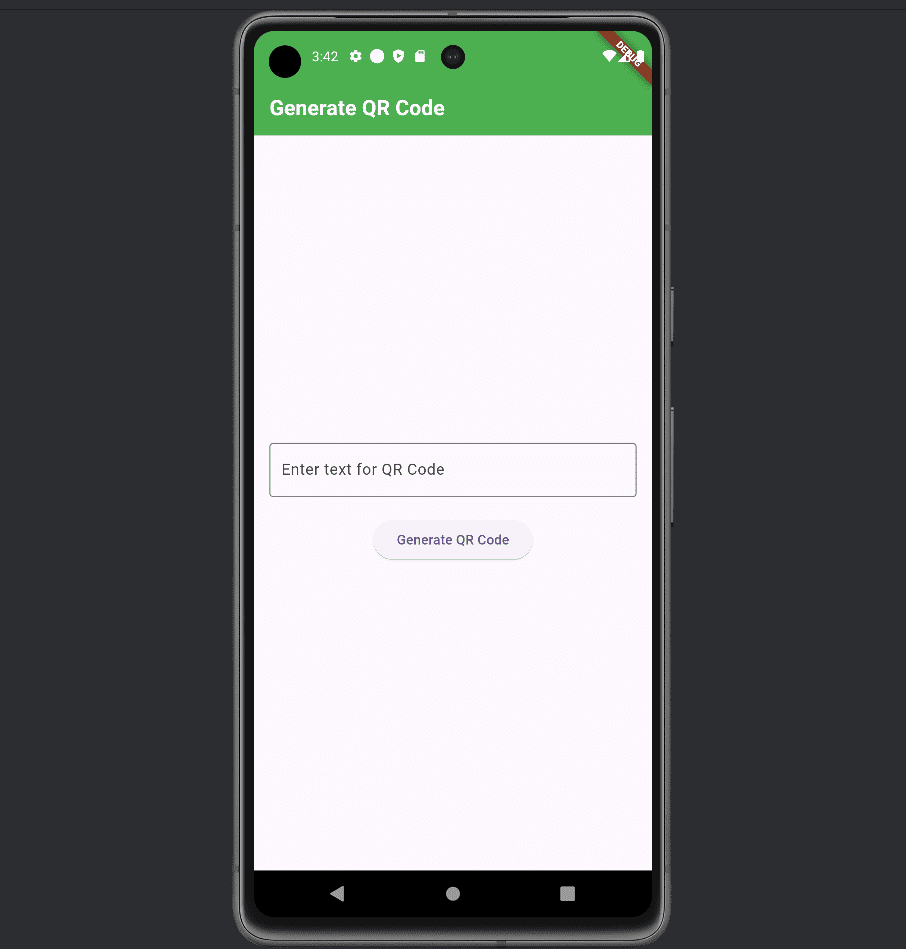
Now add a QrImageView field to the code as below:
QrImageView(
data: qrData,
size: 280,
embeddedImageStyle: const QrEmbeddedImageStyle(
size: Size(80, 80),
),
),
* QrImageView widget from qr_flutter package to generate QR codes. Displays QR code in the app.
* You can set the QR code image size (in logical pixels) using the size parameter in QrImageView.
* EmbeddedImageStyle allows you to customize the visual appearance of images embedded in QR codes. You can add a logo or small image in the middle of the QR code.
Now, want to set the qrData for the QrImageView to the value from the TextEditingController when the button is pressed:
ElevatedButton(
onPressed: () {
setState(() {
qrData = controller
.text;
});
},
child: const Text('Generate QR Code'),
),
The full code for the generated QR code is given below:
import 'package:flutter/material.dart';
import 'package:qr_flutter/qr_flutter.dart';
class QrGeneratePage extends StatefulWidget {
@override
State<QrGeneratePage> createState() => _QrGeneratePageState();
}
class _QrGeneratePageState extends State<QrGeneratePage> {
TextEditingController controller =
TextEditingController();
String qrData = "";
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Generate QR Code',
style: TextStyle(
color: Colors.white,
fontWeight: FontWeight.bold,
),
),
backgroundColor: Colors.green,
),
body: Padding(
padding: const EdgeInsets.all(16.0),
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
TextField(
controller: controller,
decoration: const InputDecoration(
labelText: 'Enter text for QR Code',
border: OutlineInputBorder(),
),
),
const SizedBox(height: 20),
ElevatedButton(
onPressed: () {
setState(() {
qrData = controller
.text;
});
},
child: const Text('Generate QR Code'),
),
const SizedBox(height: 20),
if (qrData.isNotEmpty)
QrImageView(
data: qrData,
size: 280,
embeddedImageStyle: const QrEmbeddedImageStyle(
size: Size(80, 80),
),
),
],
),
),
);
}
}
The output for the given code is provided below.
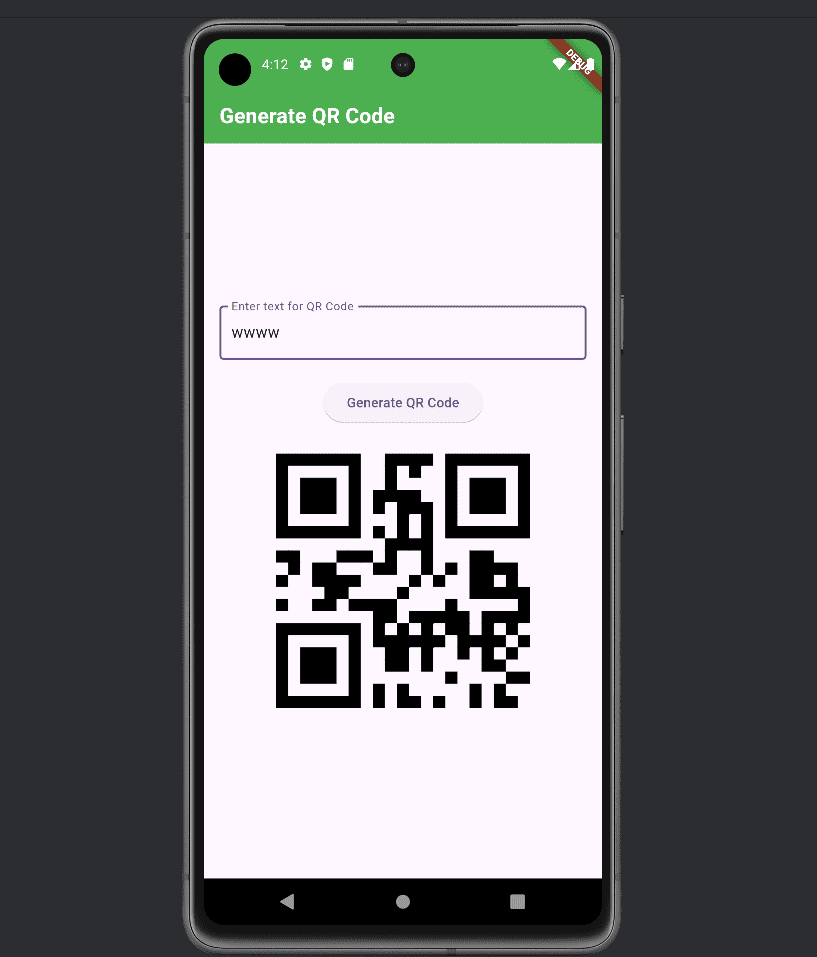
Incorporating QR code generation into your Flutter app enhances its functionality and user experience. With easy implementation, it offers a versatile way to share data and streamline interactions, making your app more dynamic and future-ready.
To read more about How to Create & Optimize Image Attachments in Flutter, refer to our blog How to Create & Optimize Image Attachments in Flutter.