In Odoo 18, the Many2Many field type allows you to create complex relationships between models, significantly enhancing data management and reporting capabilities. One of the powerful features of Odoo is the ability to group records based on Many2Many fields. This functionality enables users to analyze data more effectively by summarizing it based on related records. In this blog, we will explore how to implement and use the Group By feature for Many2Many fields in Odoo 18, including detailed code examples.
Many2Many fields in Odoo are used to define relationships where each record in one model can be associated with multiple records in another model, and vice versa. For instance, if you have a res.partner (customers) model, a Many2Many field allows each customer to have multiple tags, while each tag can be associated with multiple customers. This field type is commonly used when relationships are not exclusive and when both sides of the relationship can have multiple entries.
In practical terms, Many2Many fields improve flexibility in managing associations and are ideal for scenarios such as tags, team members in multiple projects, or categories for various products. The ability to group data by Many2Many fields further enhances reporting and data analysis by allowing users to summarize records based on shared characteristics.
Let's see how to implement this in Odoo 18 through code.
Here’s an example of using the Group By functionality with the res.partner model to group partners based on tags associated with them:
Defining the Computed Field in Python
from odoo import models, fields, api
class ResPartner(models.Model):
_inherit = 'res.partner'
tag_name = fields.Char(string='Tags Name', compute='_compute_tag_names', store=True)
@api.depends('category_id')
def _compute_tag_names(self):
for partner in self:
tag_name = ', '.join(partner.category_id.mapped('name')) if partner.category_id else ''
partner.tag_name = tag_name
XML View Definition
In your module, you should create an XML file for the view definitions. Here’s how to define a tree view for the res.partner model to include the computed tag_names field, allowing users to group by tags:
<?xml version="1.0" encoding="utf-8"?>
<odoo>
<record id="view_partner_form" model="ir.ui.view">
<field name="name">res.partner.form.inherit.custom_module</field>
<field name="model">res.partner</field>
<field name="inherit_id" ref="base.view_partner_form"/>
<field name="arch" type="xml">
<xpath expr="//field[@name='vat']" position="after">
<field name="tag_name"/>
</xpath>
</field>
</record>
<record id="view_res_partner_filter" model="ir.ui.view">
<field name="name">res.partner.search.inherit.custom_module</field>
<field name="model">res.partner</field>
<field name="inherit_id" ref="base.view_res_partner_filter"/>
<field name="arch" type="xml">
<xpath expr="//search" position="inside">
<filter name="tag_ids" string="Tags" domain="[]"
context="{'group_by': 'tag_name'}"/>
</xpath>
</field>
</record>
</odoo>
Upgrading the Module
After defining the XML view, go to the Odoo UI and upgrade the module to apply the changes. This will add the tag_names field to the form view and enable grouping by tags.
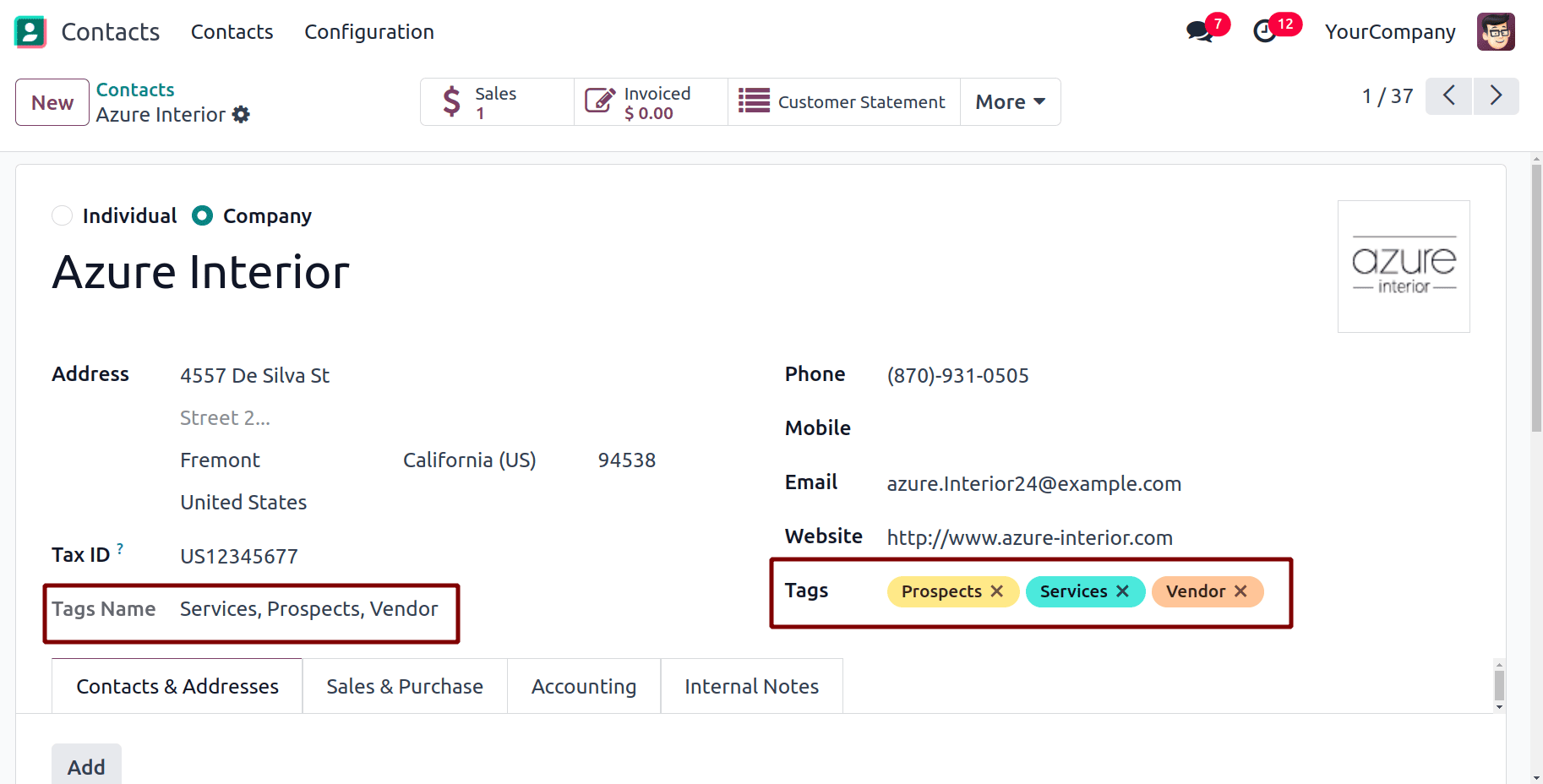
Since we added Tags in the Group By context, we can now group records based on this field in the search view.
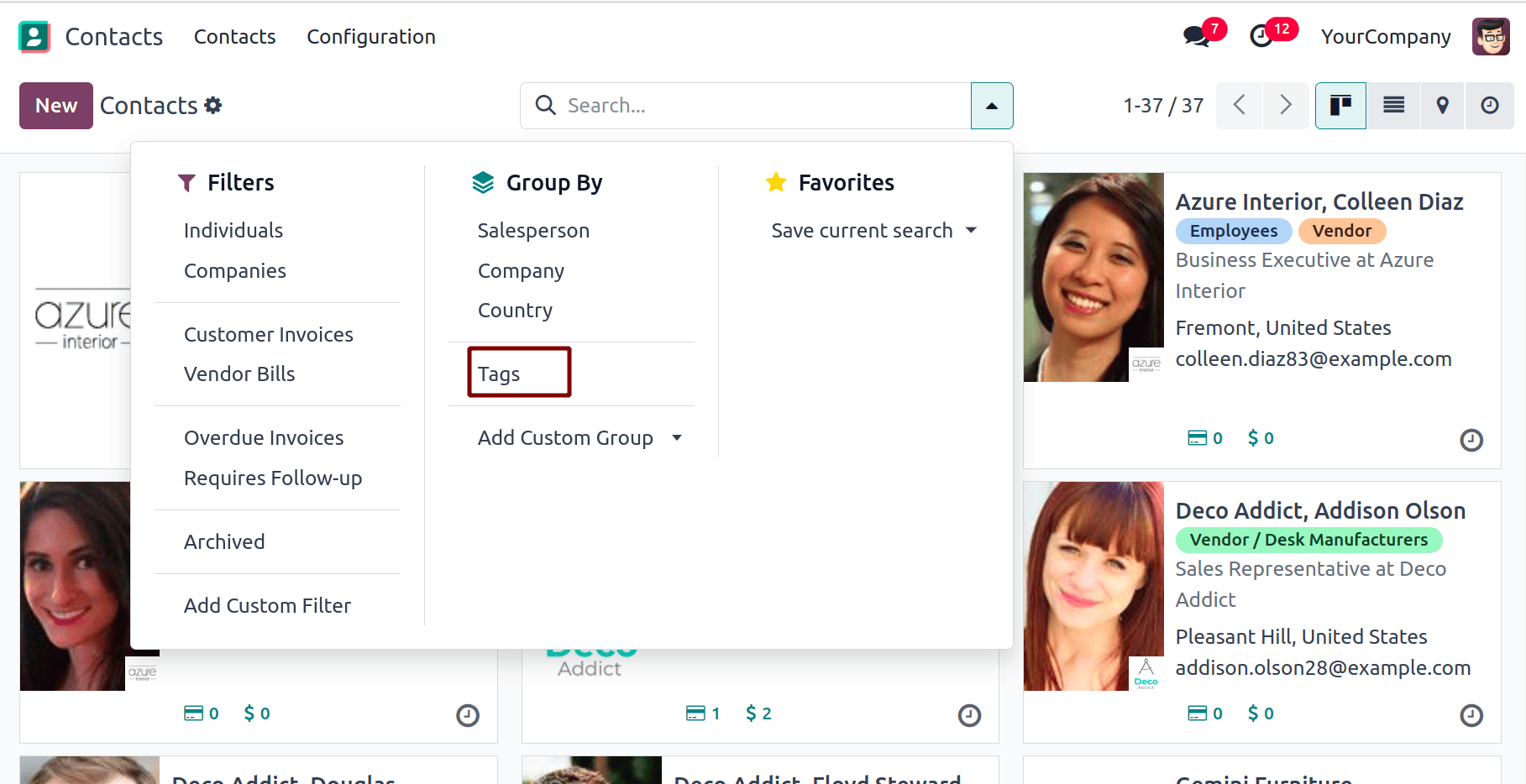
After grouping, we can see the records organized by the Many2Many field, Tags.
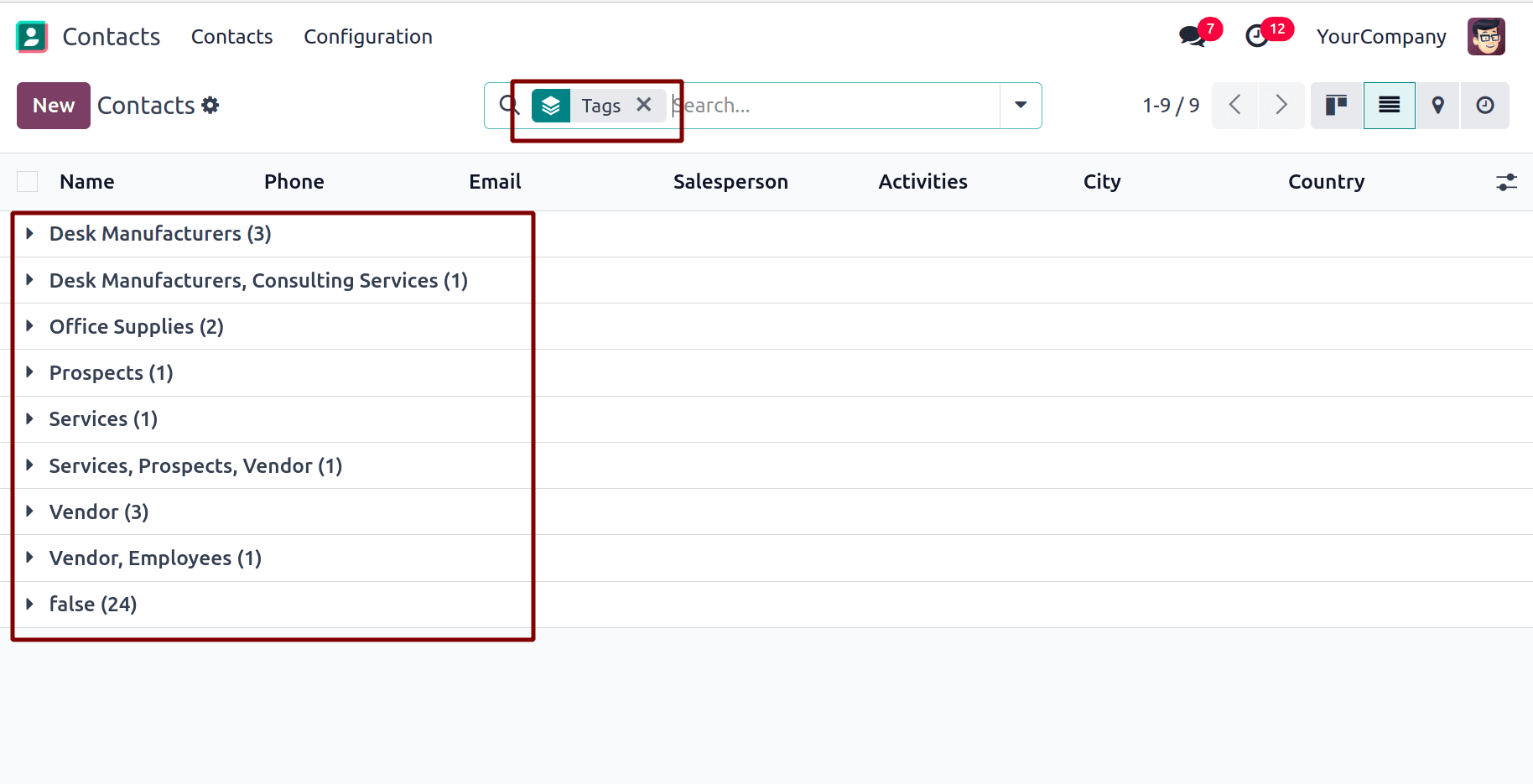
Conclusion
Using the Group By feature for Many2Many fields in Odoo 18 is straightforward and adds significant value to data reporting and analysis. By creating appropriate views and leveraging Odoo's built-in capabilities, users can easily group and analyze related records, enhancing operational efficiency.
This functionality improves data visibility and allows for better decision-making based on insights derived from grouped data. By following the steps outlined in this blog, you should be well-equipped to implement and utilize Group By functionality with Many2Many fields in your Odoo 18 applications.
To read more about How to Use Group by for Many2Many Fields in Odoo 17, refer to our blog How to Use Group by for Many2Many Fields in Odoo 17.