In this blog, we will be discussing how to set default values to fields in odoo15. In Odoo, we can set a default value for a field during the creation of a record for a model. We have many methods in odoo for setting a default value to the field. The main methods are,
1. Passing Values Through Kwargs
2.Setting value with default_get function
1. Passing Values Through Kwargs
We can set multiple kwargs for a field, which includes read-only, required, and many more. The Kwargs are keyword arguments which is a special symbol used to pass arguments. The default is kwarg, which helps set a default value for a field at the time of the creation of a model.
Let’s pass the values using default kwargs in different ways.
1.1 Set Values Directly
We can set a default value for a field like this,
state = fields.Selection([
('draft', 'Quotation'),
('sent', 'Quotation Sent'),
('sale', 'Sales Order'),
('done', 'Locked'),
('cancel', 'Canceled'),], string='Status', readonly=True,
copy=False, index=True, tracking=3, default='draft')
In this, 'state' field is a selection field and the default value of the 'state' field is set as draft i.e, Quotation.
The following screenshot shows that if the user creates a new sale order, then the default 'state' is draft, i.e., 'Quotation'.
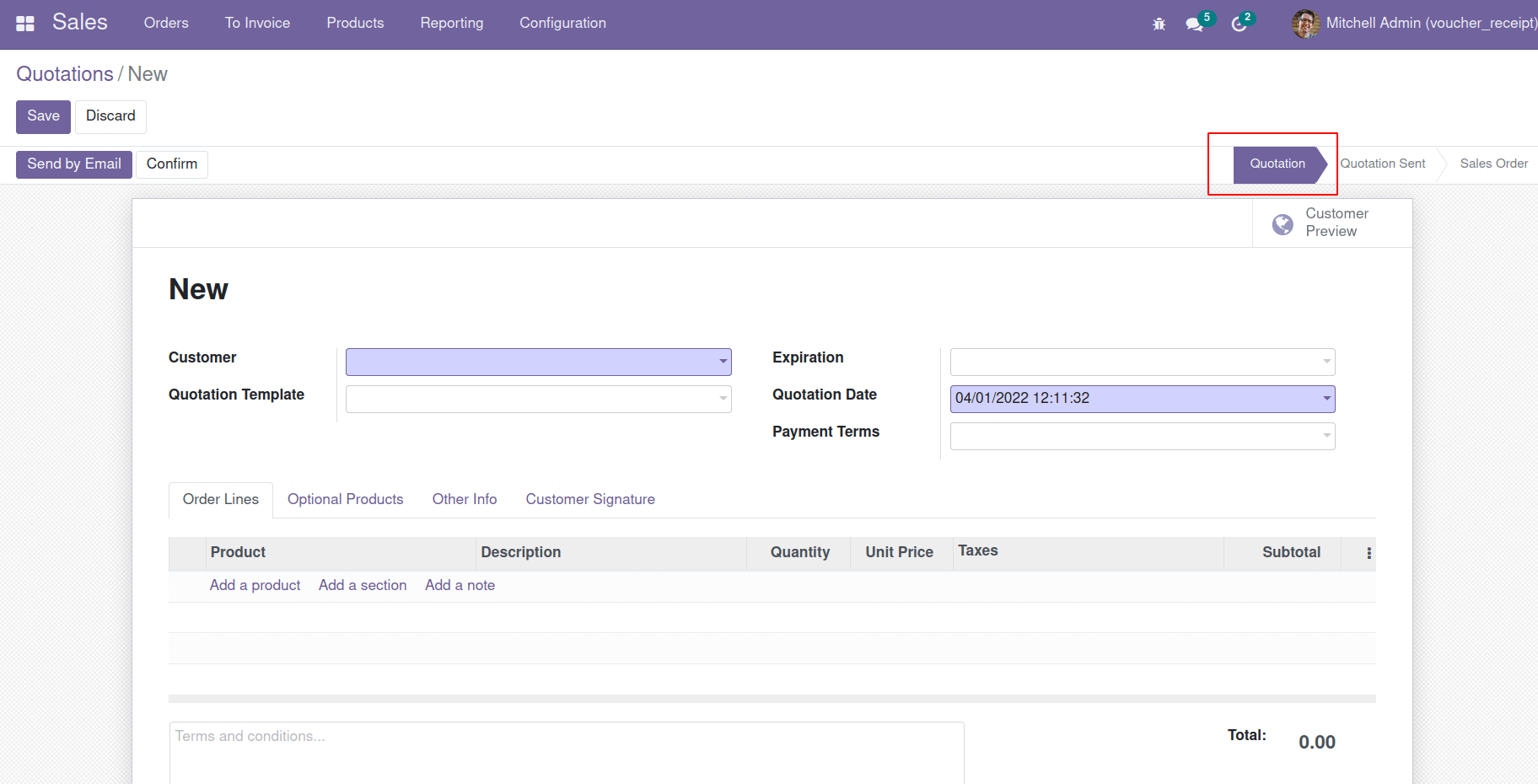
1.2 Set Values using default lambda function
A lambda function is a small anonymous function that can take any number of arguments, but it takes only one expression. The syntax of the lambda function is,
lambda arguments: expression
In odoo, the lambda function is used to return a value from the function to kwargs.
user_id = fields.Many2one(
'res.users', string='Salesperson', index=True, tracking=2,
default=lambda self: self.env.user,
domain=lambda self: "[('groups_id', '=', {}), ('share', '=', False), ('company_ids',
'=', company_id)]".format(self.env.ref("sales_team.group_sale_salesman").id
),)
In the salesperson example given above, we can see that the default value of the salesperson is set by using the lambda function. lambda self: self.env.user this lambda function is used to get the currently logged-in user. The following screenshot shows that Odoo sets the default salesperson
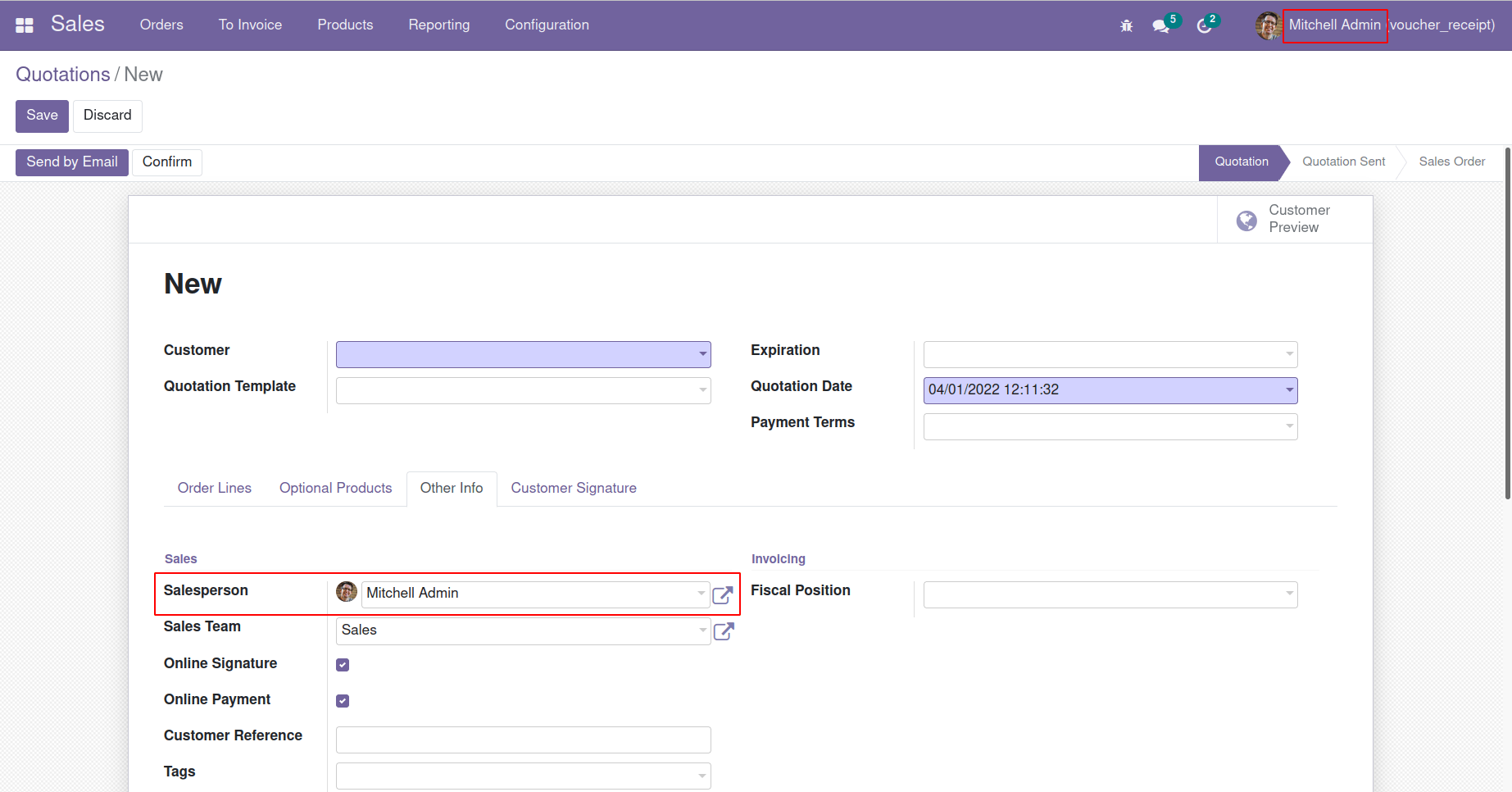
1.3 Execute a function and return values
In this method, you can set the default value of the field by executing a function. Before defining the field, the function should be called.
def _default_employee(self):
return self.env.user.employee_id
employee_id = fields.Many2one('hr.employee', string="Employee",
default=_default_employee, required=True, ondelete='cascade',
index=True)
In this above example first, define a function _default_employee() and then define a field employee_id and set default=_default_employee.
2. Setting value with default_get function
In this method, we can use a function default_get function. This function is used to set the values for multiple fields.
In the below example, we can create two new custom fields sales_person_contact and sales_person_email, and also we can see that the default values of two fields are set by using the default_get function.
class SaleOrder(models.Model):
_inherit = 'sale.order'
sales_person_contact = fields.Many2one('res.partner',
string="Salesperson Contact")
sales_person_email = fields.Char(string="Salesperson Email")
@api.model
def default_get(self, fields):
res = super(SaleOrder, self).default_get(fields)
res.update({
'sales_person_contact': self.env.user.partner_id.id or False,
'sales_person_email' : self.env.user.partner_id.email or False
})
return res
The below screenshot shows the default values of contact and email for sales person while creating a new sale order
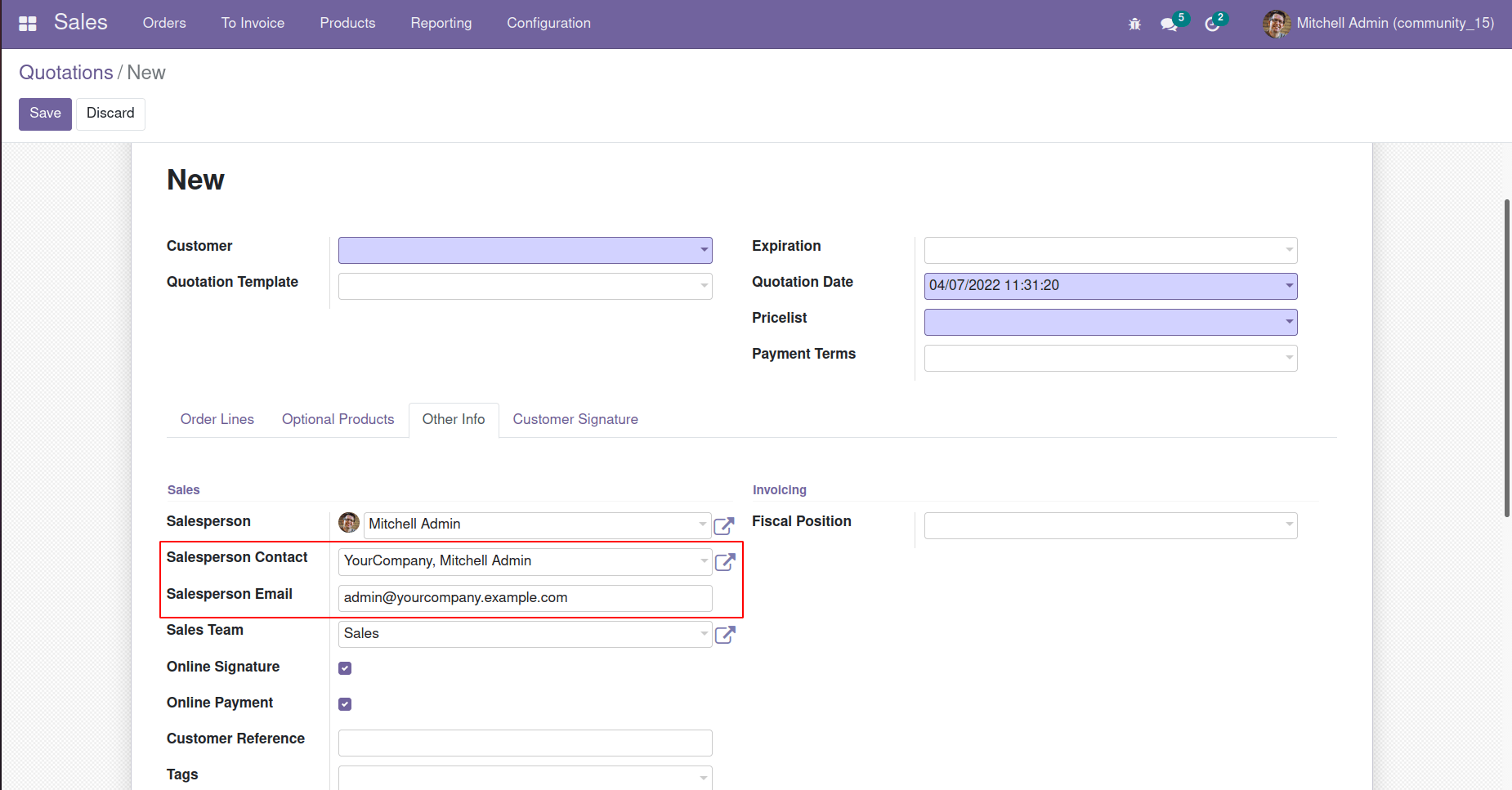
In this way we can set default values to fields in odoo15.